A class to add input neurons that can be used for custom operations. More...
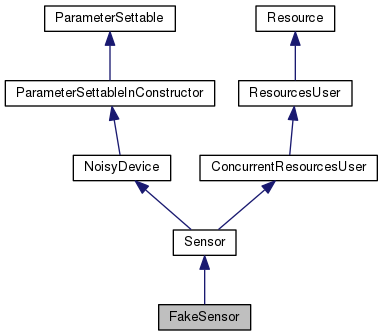
Public Member Functions | |
FakeSensor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
~FakeSensor () | |
Destructor. More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Saves the parameters of the FakeSensor into the provided ConfigurationParameters object. More... | |
virtual void | shareResourcesWith (ResourcesUser *other) |
The function to share resources. More... | |
virtual int | size () |
Returns the number of inputs. More... | |
virtual void | update () |
Updates the state of the Sensor every time step. More... | |
![]() | |
Sensor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~Sensor () | |
Destructor. More... | |
QString | name () |
Return the name of the Sensor. More... | |
void | setName (QString name) |
Use this method for changing the name of the Sensor. More... | |
![]() | |
NoisyDevice (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~NoisyDevice () | |
Destructor. More... | |
![]() | |
ParameterSettableInConstructor (ConfigurationParameters &, QString) | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
virtual void | postConfigureInitialization () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
![]() | |
void | addUsableResource (QString resource) |
void | addUsableResources (QStringList resources) |
void | declareResource (QString name, T *resource, QString lockBuddy="") |
void | deleteResource (QString name) |
T * | getResource (QString name, bool *resourceExists=NULL) |
bool | hasResource (QString name) const |
void | removeAllUsableResources () |
void | removeUsableResource (QString resource) |
void | removeUsableResources (QStringList resources) |
void | usableResources (QStringList resources) |
bool | usedResourcesExist (QStringList *nonExistingResources=NULL) const |
Static Public Member Functions | |
static void | describe (QString type) |
Describes all the parameter needed to configure this class. More... | |
![]() | |
static void | describe (QString type) |
Describe all the parameter for configuring the Sensor. More... | |
![]() | |
static void | describe (QString type) |
Describe all the parameter for configuring the Sensor. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Protected Member Functions | |
virtual void | resourceChanged (QString resourceName, ResourceChangeType changeType) |
The function called when a resource used here is changed. More... | |
![]() | |
QString | actualResourceNameForMultirobot (QString resourceName) const |
Returns the actual resource name to use. More... | |
void | checkAllNeededResourcesExist () |
Checks whether all resources we need are existing and throws an exception if they aren't. More... | |
void | resetNeededResourcesCheck () |
Resets the check on needed resources so that the next call to checkAllNeededResourcesExist() will perform the full check and not the quick one. More... | |
![]() | |
double | applyNoise (double v, double minValue, double maxValue) const |
Adds noise to the value. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
![]() | |
T * | getResource () |
![]() | |
ResourcesUser (const ResourcesUser &other) | |
virtual void | notifyResourceChange (ResourceHandler *resource, ResourceChangeType changeType)=0 |
ResourcesUser & | operator= (const ResourcesUser &other) |
Protected Attributes | |
ResourceVector< real > | m_additionalInputs |
The vector with additional inputs. More... | |
const QString | m_additionalInputsResource |
The name of the resource associated with the vector of additional inputs. More... | |
NeuronsIterator * | m_neuronsIterator |
The object to iterate over neurons of the neural network. More... | |
const QString | m_neuronsIteratorResource |
The name of th resource associated with the neural network iterator. More... | |
![]() | |
ResourceCollectionHolder | m_resources |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
typedef Resource::ResourceChangeType | ResourceChangeType |
![]() | |
enum | ResourceChangeType |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
Detailed Description
A class to add input neurons that can be used for custom operations.
This class allows to add a given number of inputs to the controller and then provides a resource to access the value of the new neurons
In addition to all parameters defined by the parent class (Sensor), this class also defines the following parameters:
- additionalInputs: the number of inputs that will be added to the controller (default 1)
- neuronsIterator: the name of the resource associated with the neural network iterator (default is "neuronsIterator")
- additionalInputsResource: the name of the resource that can be used to access the additional inputs (default is "additionalInputs")
The resources required by this Sensor are:
- name defined by the neuronsIterator parameter: the object to iterate over inputs of the controller
This sensor also defines the following resources:
- name defined by the additionalInputsResource parameter: the name of the resource that can be used to access the additional inputs. This can be accessed as a farsa::ResourceVector<real>
Constructor & Destructor Documentation
FakeSensor | ( | ConfigurationParameters & | params, |
QString | prefix | ||
) |
Constructor.
- Parameters
-
params the ConfigurationParameters containing the parameters prefix the path prefix to the paramters for this Motor
Definition at line 42 of file sensors.cpp.
References FakeSensor::m_additionalInputs, FakeSensor::m_additionalInputsResource, FakeSensor::m_neuronsIteratorResource, ResourceVector< class >::size(), and ConcurrentResourcesUser::usableResources().
~FakeSensor | ( | ) |
Destructor.
Definition at line 56 of file sensors.cpp.
References ConcurrentResourcesUser::deleteResource(), and FakeSensor::m_additionalInputsResource.
Member Function Documentation
|
static |
Describes all the parameter needed to configure this class.
- Parameters
-
type a string representation for the name of this type
Definition at line 75 of file sensors.cpp.
References ParameterSettable::addTypeDescription(), Sensor::describe(), and ParameterSettable::IsMandatory.
|
protectedvirtual |
The function called when a resource used here is changed.
- Parameters
-
resourceName the name of the resource that has changed. chageType the type of change the resource has gone through (whether it was created, modified or deleted)
Reimplemented from ConcurrentResourcesUser.
Definition at line 113 of file sensors.cpp.
References Logger::info(), FakeSensor::m_additionalInputsResource, FakeSensor::m_neuronsIterator, FakeSensor::m_neuronsIteratorResource, Sensor::name(), NeuronsIterator::nextNeuron(), Sensor::resetNeededResourcesCheck(), NeuronsIterator::setCurrentBlock(), NeuronsIterator::setGraphicProperties(), and FakeSensor::size().
|
virtual |
Saves the parameters of the FakeSensor into the provided ConfigurationParameters object.
- Parameters
-
params the ConfigurationParameters where save the parameters prefix the path prefix for the parameters to save
Reimplemented from Sensor.
Definition at line 66 of file sensors.cpp.
References ConfigurationParameters::createParameter(), FakeSensor::m_additionalInputs, FakeSensor::m_additionalInputsResource, FakeSensor::m_neuronsIteratorResource, Sensor::save(), ResourceVector< class >::size(), and ConfigurationParameters::startObjectParameters().
|
virtual |
The function to share resources.
The calling instance will lose the possibility to access the resources it had before this call
- Parameters
-
other the instance with which resources will be shared. If NULL we lose the association with other objects and start with an empty resource set
- Note
- This is NOT thread safe (both this and the other instance should not be being accessed by other threads)
Reimplemented from ConcurrentResourcesUser.
Definition at line 104 of file sensors.cpp.
References ConcurrentResourcesUser::declareResource(), FakeSensor::m_additionalInputs, FakeSensor::m_additionalInputsResource, and ConcurrentResourcesUser::shareResourcesWith().
|
virtual |
Returns the number of inputs.
This returns the value set for the paramenter additionalInputs
Implements Sensor.
Definition at line 99 of file sensors.cpp.
References FakeSensor::m_additionalInputs, and ResourceVector< class >::size().
Referenced by FakeSensor::resourceChanged().
|
virtual |
Updates the state of the Sensor every time step.
Implements Sensor.
Definition at line 85 of file sensors.cpp.
References Sensor::checkAllNeededResourcesExist(), FakeSensor::m_additionalInputs, FakeSensor::m_neuronsIterator, Sensor::name(), NeuronsIterator::nextNeuron(), NeuronsIterator::setCurrentBlock(), NeuronsIterator::setInput(), and ResourceVector< class >::size().
Member Data Documentation
|
protected |
The vector with additional inputs.
Definition at line 133 of file sensors.h.
Referenced by FakeSensor::FakeSensor(), FakeSensor::save(), FakeSensor::shareResourcesWith(), FakeSensor::size(), and FakeSensor::update().
|
protected |
The name of the resource associated with the vector of additional inputs.
Definition at line 145 of file sensors.h.
Referenced by FakeSensor::FakeSensor(), FakeSensor::resourceChanged(), FakeSensor::save(), FakeSensor::shareResourcesWith(), and FakeSensor::~FakeSensor().
|
protected |
The object to iterate over neurons of the neural network.
Definition at line 150 of file sensors.h.
Referenced by FakeSensor::resourceChanged(), and FakeSensor::update().
|
protected |
The name of th resource associated with the neural network iterator.
Definition at line 139 of file sensors.h.
Referenced by FakeSensor::FakeSensor(), FakeSensor::resourceChanged(), and FakeSensor::save().
The documentation for this class was generated from the following files:
- experiments/include/sensors.h
- experiments/src/sensors.cpp