The base abstract class for the Motor hierarchy. More...
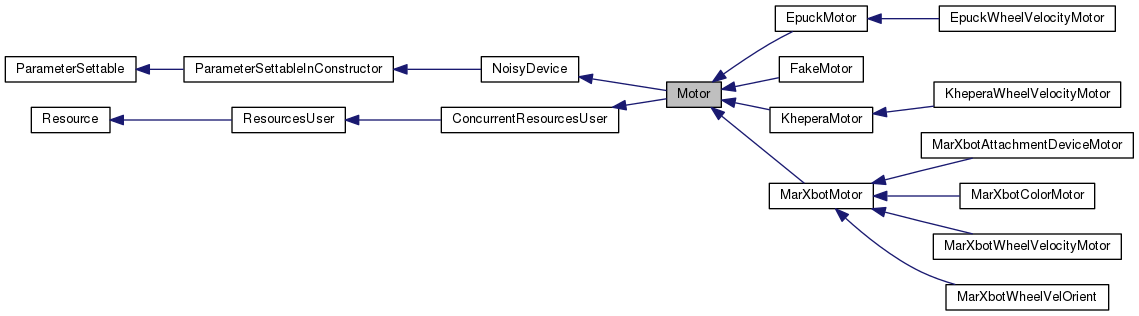
Public Member Functions | |
Motor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~Motor () | |
Destructor. More... | |
QString | name () |
Return the name of the Sensor. More... | |
void | save (ConfigurationParameters ¶ms, QString prefix) |
Save the parameters into the ConfigurationParameters. More... | |
void | setName (QString name) |
Use this method for changing the name of the Sensor. More... | |
virtual int | size ()=0 |
Return the number of neurons from which the Motor will get the output. More... | |
virtual void | update ()=0 |
Update the state of the Motor every time step; the actual behaviour is implemented in subclasses. More... | |
![]() | |
NoisyDevice (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~NoisyDevice () | |
Destructor. More... | |
![]() | |
ParameterSettableInConstructor (ConfigurationParameters &, QString) | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
virtual void | postConfigureInitialization () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
![]() | |
void | addUsableResource (QString resource) |
void | addUsableResources (QStringList resources) |
void | declareResource (QString name, T *resource, QString lockBuddy="") |
void | deleteResource (QString name) |
T * | getResource (QString name, bool *resourceExists=NULL) |
bool | hasResource (QString name) const |
void | removeAllUsableResources () |
void | removeUsableResource (QString resource) |
void | removeUsableResources (QStringList resources) |
virtual void | shareResourcesWith (ResourcesUser *buddy) |
void | usableResources (QStringList resources) |
bool | usedResourcesExist (QStringList *nonExistingResources=NULL) const |
Static Public Member Functions | |
static void | describe (QString type) |
Describe all the parameter for configuring the Motor. More... | |
![]() | |
static void | describe (QString type) |
Describe all the parameter for configuring the Sensor. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Protected Member Functions | |
QString | actualResourceNameForMultirobot (QString resourceName) const |
Returns the actual resource name to use. More... | |
void | checkAllNeededResourcesExist () |
Checks whether all resources we need are existing and throws an exception if they aren't. More... | |
void | resetNeededResourcesCheck () |
Resets the check on needed resources so that the next call to checkAllNeededResourcesExist() will perform the full check and not the quick one. More... | |
![]() | |
double | applyNoise (double v, double minValue, double maxValue) const |
Adds noise to the value. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
![]() | |
T * | getResource () |
virtual void | resourceChanged (QString name, ResourceChangeType changeType) |
![]() | |
ResourcesUser (const ResourcesUser &other) | |
virtual void | notifyResourceChange (ResourceHandler *resource, ResourceChangeType changeType)=0 |
ResourcesUser & | operator= (const ResourcesUser &other) |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
typedef Resource::ResourceChangeType | ResourceChangeType |
![]() | |
enum | ResourceChangeType |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
![]() | |
ResourceCollectionHolder | m_resources |
Detailed Description
The base abstract class for the Motor hierarchy.
A Motor is an object that read data from the neural network and put that data into a resource converting it into the appropriate way. This class inherits from NoisyDevice, so you can apply noise using the applyNoise() function. In child class implement also the resourceChanged() function to get notified of resources creation/modification
Definition at line 272 of file neuroninterfaces.h.
Constructor & Destructor Documentation
Motor | ( | ConfigurationParameters & | params, |
QString | prefix | ||
) |
Constructor and Configure.
- Parameters
-
params is the ConfigurationParameters containing the parameters prefix is the path prefix to the paramters for this Motor
Definition at line 190 of file neuroninterfaces.cpp.
References ConfigurationHelper::getString(), and Motor::setName().
~Motor | ( | ) |
Destructor.
Definition at line 198 of file neuroninterfaces.cpp.
Member Function Documentation
|
protected |
Returns the actual resource name to use.
This function mangles the name of the resource so that the correct name is used in case of multirobot setups. NOTE: use this only for resources that are robot-specific because the name is always mangled (no check is done to see if resourceName is already the name of an existing resource)
Definition at line 251 of file neuroninterfaces.cpp.
Referenced by EpuckMotor::EpuckMotor(), KheperaMotor::KheperaMotor(), and MarXbotMotor::MarXbotMotor().
|
protected |
Checks whether all resources we need are existing and throws an exception if they aren't.
The result of the check is stored, so subsequent calls to this functions are quick (unless you call resetNeededResourcesCheck(), see that function description)
Definition at line 231 of file neuroninterfaces.cpp.
References ConfigurationHelper::throwUserMissingResourceError(), and ConcurrentResourcesUser::usedResourcesExist().
Referenced by FakeMotor::update(), MarXbotWheelVelocityMotor::update(), KheperaWheelVelocityMotor::update(), EpuckWheelVelocityMotor::update(), MarXbotWheelVelOrient::update(), MarXbotColorMotor::update(), and MarXbotAttachmentDeviceMotor::update().
|
static |
Describe all the parameter for configuring the Motor.
Definition at line 214 of file neuroninterfaces.cpp.
References ParameterSettable::addTypeDescription(), and NoisyDevice::describe().
Referenced by EpuckMotor::describe(), KheperaMotor::describe(), MarXbotMotor::describe(), and FakeMotor::describe().
QString name | ( | ) |
Return the name of the Sensor.
Typically, it is the name of the subclass
Definition at line 223 of file neuroninterfaces.cpp.
Referenced by FakeMotor::resourceChanged(), Motor::setName(), FakeMotor::update(), MarXbotWheelVelocityMotor::update(), KheperaWheelVelocityMotor::update(), EpuckWheelVelocityMotor::update(), MarXbotWheelVelOrient::update(), MarXbotColorMotor::update(), and MarXbotAttachmentDeviceMotor::update().
|
protected |
Resets the check on needed resources so that the next call to checkAllNeededResourcesExist() will perform the full check and not the quick one.
Call this if you get notified that a resource has been deleted so that we can check if it is available again when you need it
Definition at line 246 of file neuroninterfaces.cpp.
Referenced by EpuckMotor::resourceChanged(), KheperaMotor::resourceChanged(), MarXbotMotor::resourceChanged(), and FakeMotor::resourceChanged().
|
virtual |
Save the parameters into the ConfigurationParameters.
- Parameters
-
params is the ConfigurationParameters where save the parameters prefix is the path prefix for the parameters to save
Reimplemented from NoisyDevice.
Definition at line 201 of file neuroninterfaces.cpp.
References ConfigurationParameters::createParameter(), NoisyDevice::save(), and ConfigurationParameters::startObjectParameters().
Referenced by EpuckMotor::save(), KheperaMotor::save(), MarXbotMotor::save(), and FakeMotor::save().
void setName | ( | QString | name | ) |
Use this method for changing the name of the Sensor.
Definition at line 227 of file neuroninterfaces.cpp.
References Motor::name().
Referenced by Motor::Motor().
|
pure virtual |
Return the number of neurons from which the Motor will get the output.
- Warning
- this method has to be called in any situation; when re-implement it does not rely on conditions/variables available only after the initilization because this method might be called before the Motor initialization
Implemented in MarXbotAttachmentDeviceMotor, MarXbotColorMotor, MarXbotWheelVelOrient, EpuckWheelVelocityMotor, KheperaWheelVelocityMotor, MarXbotWheelVelocityMotor, and FakeMotor.
|
pure virtual |
Update the state of the Motor every time step; the actual behaviour is implemented in subclasses.
Implemented in MarXbotAttachmentDeviceMotor, MarXbotColorMotor, MarXbotWheelVelOrient, EpuckWheelVelocityMotor, KheperaWheelVelocityMotor, MarXbotWheelVelocityMotor, and FakeMotor.
The documentation for this class was generated from the following files:
- experiments/include/neuroninterfaces.h
- experiments/src/neuroninterfaces.cpp