The base class for experiments. More...
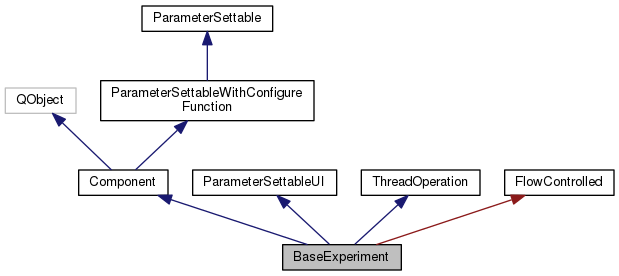
Classes | |
class | AbstractOperationWrapper |
The base abstract class for operation wrappers. More... | |
class | OperationWrapper |
The class for operation wrappers. More... | |
class | OperationWrapperOneParameter |
The class for operation wrappers taking one parameter. More... | |
class | OperationWrapperThreeParameters |
The class for operation wrappers taking three parameters. More... | |
class | OperationWrapperTwoParameters |
The class for operation wrappers taking two parameters. More... | |
Public Slots | |
virtual void | stopCurrentOperation (bool wait) |
Forces the end of the current operation, if threaded. More... | |
virtual void | stopCurrentOperation () |
Forces the end of the current operation, if threaded. More... | |
Public Member Functions | |
BaseExperiment () | |
Constructor. More... | |
virtual | ~BaseExperiment () |
Destructor. More... | |
virtual void | addAdditionalMenus (QMenuBar *menuBar) |
Adds additional menus to the menu bar of Total99. More... | |
void | changeInterval (unsigned long interval) |
Changes the delay between subsequent steps of a steppable operation. More... | |
virtual void | configure (ConfigurationParameters ¶ms, QString prefix) |
Configures the object using a ConfigurationParameters object. More... | |
unsigned long | currentInterval () const |
Returns the current delay for steppable operations. More... | |
virtual void | fillActionsMenu (QMenu *actionsMenu) |
Fills the menu "Actions" of Total99. More... | |
FlowController * | flowController () |
Returns the flow controller. More... | |
const QVector< AbstractOperationWrapper * > & | getOperations () const |
Returns the list of operations. More... | |
virtual ParameterSettableUI * | getUIManager () |
Returns an instance of the class handling our viewers. More... | |
DataUploaderDownloader< __BaseExperiment_internal::OperationStatus, __BaseExperiment_internal::OperationControl > * | getUploaderDownloader () |
Returns the object to exchange data. More... | |
virtual QList< ParameterSettableUIViewer > | getViewers (QWidget *parent, Qt::WindowFlags flags) |
Returns the list of viewers. More... | |
void | pause () |
Puts the current operation in pause. More... | |
virtual void | postConfigureInitialization () |
This function is called after all linked objects have been configured. More... | |
void | resume () |
Resumes the current operation if paused. More... | |
virtual void | run () |
Runs the next operation. More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Saves the actual status of parameters into the ConfigurationParameters object passed. More... | |
void | step () |
Performs a single step for the current steppable operation. More... | |
virtual void | stop () |
Forces the end of the experiment. More... | |
![]() | |
Component () | |
Constructor. More... | |
virtual | ~Component () |
Destructor. More... | |
void | setStatus (QString newStatus) |
used by subclasses to change the status of the experiment More... | |
QString | status () |
return a text description of the current status of the component More... | |
virtual void | stopCurrentOperation ()=0 |
Reimplement in subclasses to stop the running operation. More... | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
Static Public Member Functions | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Protected Member Functions | |
template<class T > | |
void | addOperation (QString name, void(T::*func)(), bool useSeparateThread, bool steppable) |
The function that adds the given operation to the list of all operations declared by the experiment. More... | |
template<class T , class P0 > | |
void | addOperation (QString name, void(T::*func)(P0), P0 p0, bool useSeparateThread, bool steppable) |
The function that adds the given operation that takes one parameter to the list of all operations declared by the experiment. More... | |
template<class T , class P0 , class P1 > | |
void | addOperation (QString name, void(T::*func)(P0, P1), P0 p0, P1 p1, bool useSeparateThread, bool steppable) |
The function that adds the given operation that takes two parameters to the list of all operations declared by the experiment. More... | |
template<class T , class P0 , class P1 , class P2 > | |
void | addOperation (QString name, void(T::*func)(P0, P1, P2), P0 p0, P1 p1, P2 p2, bool useSeparateThread, bool steppable) |
The function that adds the given operation that takes three parameters to the list of all operations declared by the experiment. More... | |
bool | batchRunning () const |
Returns true if we are running in batch. More... | |
QList< QAction * > | getActionsForOperations (QObject *actionsParent) const |
Returns a list of actions, one for each operation declared by the experiment. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
Friends | |
class | BaseExperimentFlowController |
The BaseExperimentFlowController class is friend to access our private members and functions. More... | |
class | Notifee |
The Notifee class is friend to access our private members and functions. More... | |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
void | statusChanged (QString newStatus) |
emitted when the status is changed More... | |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
Detailed Description
The base class for experiments.
Use this class as parent of experiments. This only provides some helper code to run operations in a worker thread and to add menus and guis to Total99. By using this you don't need to create a component and uimanager separately and you also have some ready-to-use code that supports different operations. When using this class you have to define which actions the class provides and to implement the function which execute the actions as QT slots. The code in this class takes care of adding the actions to Total99 menus and of starting the functions in a worker thread if they are supposed to. To add operations you can either call addOperation() or use the DECLARE_THREAD_OPERATION, DECLARE_STEPPABLE_THREAD_OPERATION or DECLARE_IMMEDIATE_OPERATION macro (see below for more information) and then implement the slot with the operation. Both addOperation and the macros should be called in the experiment constructor, in the configure method or in the postConfigureInitialization method (because the list of operations is generally asked after these functions are called and there is no way to send notifications if the list changes). Here is an example:
The difference between using the addOperation() function and the macros is that the function lets you define a custom name for the operation to be used in the Actions menĂ¹ of Total99 but has a slightly more complex sintax, while the macros have a clearer syntax at the expense of some flexibility. Moreover the addOperation() function has overloads to define operations that take one or more parameters, binding the parameters to fixed values (see the description of the addOperation function). As you can see from the example above, your experiment must inherit from BaseExperiment. The Experiment class must have a default constructor and the Q_OBJECT macro must be present. This class always adds a stop operation to stop the current actions as the first operation in the list. Operations can run in three possible modalities, each corresponding to one of the DECLARE_*_OPERATION macro (see the description of the addOperation() function for information about which parameters to use to obtain the various execution modalities):
- run operation in a different thread (DECLARE_THREAD_OPERATION). In this case the function associated with the operation is run in a thread different from the one in which the BaseExperiment subclass instance lives (in Total99 components like BaseExperiment live in the GUI thread). This means that care has to be taken when exchanging data with the GUI (e.g. using the facilities of the classes described in dataexchange.h), but also that the operation doesn't lock the GUI thread, When implementing this kind of operations, the stopFlow() function should be called regularly to check if a premature end of the operation has been requested;
- run operation in a different thread and provide the possibility to run step-by-step (DECLARE_STEPPABLE_THREAD_OPERATION). This is the same as the modality above (DECLARE_THREAD_OPERATION) with the additional possiblity to pause the operation, perform single steps or put a delay between two consecutive steps. How steps are defined is left to the implementation: a step is the portion of code between two subsequent calls to the pauseFlow() function;
- run operation immediately (DECLARE_IMMEDIATE_OPERATION). In this case the operation is run in the same thread of the BaseExperiment subclass instance. This means that the execution of the operation is like a classical function call: if the object lives in the GUI thread the GUI will be non-responsive until the operation has finished executing. Because of this, this modality should be used only for short operations The stopFlow() and pauseFlow() functions are present because this inherits FlowControlled (they are protected functions of this class, even if FlowControlled itself is inherited as private to disallow changing the FlowController). This class also provides a public function flowController() that returns the FlowController object used by this class (that can be passed to other objects to share the same control logic). If the experiment needs one or more GUIs you can re-implement the getViewers() function (which is the same as ParameterSettableUI::getViewers) to return the list of available widgets. The default implementation of this function creates BaseExperimentGUI to control the base experiment execution, so if you want that GUI remember to call BaseExperiment::getViewers() when reimplementing that function. If more menus are required, the experiment can re-implement the addAdditionalMenus() function (which is the same as ParameterSettableUI::addAdditionalMenus())
- Note
- Most of the functions of this class are NOT thread-safe, so you should not call them from slots implementing thread operations. If a function is thread safe, the function documentation states it explicitly
- When in subclasses you have threaded operations, you must be sure that no operation is running (e.g. by calling stopCurrentOperation()) before destroying the object. In fact the thread is stopped in BaseExperiment destructor, but subclasses destructor is called before the one of BaseExperiment, so you could end up deleting stuffs while an operation is still running.
Definition at line 265 of file baseexperiment.h.
Constructor & Destructor Documentation
BaseExperiment | ( | ) |
Constructor.
Definition at line 157 of file baseexperiment.cpp.
References BaseExperiment::addOperation(), DataUploader< DataType_t >::checkAssociationBeforeUpload(), FlowControlled::setFlowController(), and BaseExperiment::stopCurrentOperation().
|
virtual |
Destructor.
Before destroyng subclasses, you must be sure no operation is running. You can call stopCurrentOperation() to make sure this is true
Definition at line 191 of file baseexperiment.cpp.
Member Function Documentation
|
virtual |
Adds additional menus to the menu bar of Total99.
- Parameters
-
menuBar the menu bar of the Total99 application
Reimplemented from ParameterSettableUI.
Definition at line 254 of file baseexperiment.cpp.
Referenced by BaseExperimentUIManager::addAdditionalMenus().
|
inlineprotected |
The function that adds the given operation to the list of all operations declared by the experiment.
This function should be called in the constructor, in the configre method or in the postConfigureInitialization method to declare all operations provided by the class. The DECLARE_*_OPERATION macros simply call this functions with the proper parameters. Here is the correspondence between parameters of this functions and the DECLARE_*_OPERATION macros:
- useSeparateThread = false is like using the DECLARE_IMMEDIATE_OPERATION
- useSeparateThread = true AND steppable = false is like using DECLARE_THREAD_OPERATION
- useSeparateThread = true AND steppable = true is like using DECLARE_STEPPABLE_THREAD_OPERATION
- Parameters
-
name the name of the operations func the function implementing the operation (which should be a slot) useSeparateThread if true the action is run in a separate thread, if false it is run in the thread of the caller steppable if useSeparateThread is true and this is also true, the operation is steppable, if useSeparateThread is false this value is ignored.
Definition at line 1035 of file baseexperiment.h.
References OperationStatus::NewOperation.
Referenced by BaseExperiment::BaseExperiment().
|
inlineprotected |
The function that adds the given operation that takes one parameter to the list of all operations declared by the experiment.
This is an overload that allows using a function that takes one parameter as an operation by binding the parameter to a given value (passed as the p0 parameter to this function)
- Parameters
-
name the name of the operations func the function implementing the operation (which should be a slot) p0 the value of the parameter of the function useSeparateThread if true the action is run in a separate thread, if false it is run in the thread of the caller steppable if useSeparateThread is true and this is also true, the operation is steppable, if useSeparateThread is false this value is ignored.
Definition at line 1068 of file baseexperiment.h.
References OperationStatus::NewOperation.
|
inlineprotected |
The function that adds the given operation that takes two parameters to the list of all operations declared by the experiment.
This is an overload that allows using a function that takes two parameters as an operation by binding the parameters to given values (passed as the p0 and p1 parameters to this function)
- Parameters
-
name the name of the operations func the function implementing the operation (which should be a slot) p0 the value of the first parameter of the function p1 the value of the second parameter of the function useSeparateThread if true the action is run in a separate thread, if false it is run in the thread of the caller steppable if useSeparateThread is true and this is also true, the operation is steppable, if useSeparateThread is false this value is ignored.
Definition at line 1102 of file baseexperiment.h.
References OperationStatus::NewOperation.
|
inlineprotected |
The function that adds the given operation that takes three parameters to the list of all operations declared by the experiment.
This is an overload that allows using a function that takes three parameters as an operation by binding the parameters to given values (passed as the p0, p1 and p2 parameters to this function)
- Parameters
-
name the name of the operations func the function implementing the operation (which should be a slot) p0 the value of the first parameter of the function p1 the value of the second parameter of the function p2 the value of the third parameter of the function useSeparateThread if true the action is run in a separate thread, if false it is run in the thread of the caller steppable if useSeparateThread is true and this is also true, the operation is steppable, if useSeparateThread is false this value is ignored.
Definition at line 1137 of file baseexperiment.h.
References OperationStatus::NewOperation.
|
protected |
Returns true if we are running in batch.
- Returns
- true if we are running in batch
Definition at line 367 of file baseexperiment.cpp.
void changeInterval | ( | unsigned long | interval | ) |
Changes the delay between subsequent steps of a steppable operation.
This only affects steppable operations. The default value of the delay is 0
- Parameters
-
interval the new delay in milliseconds
- Note
- this function is thread-safe
Definition at line 316 of file baseexperiment.cpp.
|
virtual |
Configures the object using a ConfigurationParameters object.
- Parameters
-
params the configuration parameters object with parameters to use prefix the prefix to use to access the object configuration parameters. This is guaranteed to end with the separator character when called by the factory, so you don't need to add one
Implements Component.
Definition at line 202 of file baseexperiment.cpp.
References ConfigurationHelper::getBool().
unsigned long currentInterval | ( | ) | const |
Returns the current delay for steppable operations.
- Returns
- the current delay for steppable operations
Definition at line 321 of file baseexperiment.cpp.
Referenced by BaseExperimentGUI::BaseExperimentGUI().
|
static |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups.
It's mandatory in all subclasses where configure and save methods have been re-implemented for dealing with new parameters and subgroups to also implement the describe method
- Parameters
-
type is the name of the type regarding the description. The type is used when a subclass reuse the description of its parent calling the parent describe method passing the type of the subclass. In this way, the result of the method describe of the parent will be the addition of the description of the parameters of the parent class into the type of the subclass
Definition at line 215 of file baseexperiment.cpp.
References ParameterSettable::addTypeDescription().
|
virtual |
Fills the menu "Actions" of Total99.
This function takes care of filling the menu "Actions" of Total99 with the actions declared by Experiment
- Parameters
-
actionsMenu the "Actions" menu in Total99
- Note
- You should not override this function unless you have particular needings
Reimplemented from ParameterSettableUI.
Definition at line 233 of file baseexperiment.cpp.
References BaseExperiment::getActionsForOperations().
Referenced by BaseExperimentUIManager::fillActionsMenu().
|
inline |
Returns the flow controller.
You can use it with other objects so that they share the same control strategy of this class
- Returns
- the flow controller used by this class. You MUST not delete it!
Definition at line 952 of file baseexperiment.h.
|
protected |
Returns a list of actions, one for each operation declared by the experiment.
This function can be used if you decide to re-implement the fillActionsMenu function to obtain the list of actions without filling the menu
- Parameters
-
actionsParent the parent of generated QActions
- Returns
- The list of actions, one for each operation declared by the experiment
Definition at line 347 of file baseexperiment.cpp.
Referenced by BaseExperiment::fillActionsMenu().
const QVector< BaseExperiment::AbstractOperationWrapper * > & getOperations | ( | ) | const |
Returns the list of operations.
- Returns
- the list of operations
Definition at line 326 of file baseexperiment.cpp.
|
virtual |
Returns an instance of the class handling our viewers.
This returns a pointer to the ParameterSettableUI object. That object simply calls functions in this class
- Returns
- a pointer to the ParameterSettableUI object
- Note
- You should not override this function
Reimplemented from ParameterSettable.
Definition at line 228 of file baseexperiment.cpp.
DataUploaderDownloader< __BaseExperiment_internal::OperationStatus, __BaseExperiment_internal::OperationControl > * getUploaderDownloader | ( | ) |
Returns the object to exchange data.
This is public so that it is possible to use a custom GUI (in that case the getViewers() function must be overridden to prevent returning a BaseExperimentGUI)
- Returns
- a pointer to the object to exchange data
Definition at line 331 of file baseexperiment.cpp.
Referenced by BaseExperimentGUI::BaseExperimentGUI().
|
virtual |
Returns the list of viewers.
Override this function to return the list of all viewers for this experiment. The default implementation adds the BaseExperimentGUI widget, so call this function in subclasses if you want that window
- Parameters
-
parent the parent widget for all viewers created by this function flags the window flags to specify when constructing the widgets
- Returns
- the list of widgets
Reimplemented from ParameterSettableUI.
Definition at line 243 of file baseexperiment.cpp.
Referenced by BaseExperimentUIManager::getViewers().
void pause | ( | ) |
Puts the current operation in pause.
This only works for steppable operations
- Note
- this function is thread-safe
Definition at line 286 of file baseexperiment.cpp.
|
virtual |
This function is called after all linked objects have been configured.
See the description of the ConfigurationParameters class for more information. This starts the inner thread
Reimplemented from ParameterSettable.
Definition at line 220 of file baseexperiment.cpp.
References Component::setStatus().
void resume | ( | ) |
Resumes the current operation if paused.
The operation must be paused, otherwise this function does nothing
- Note
- this function is thread-safe
Definition at line 303 of file baseexperiment.cpp.
|
virtual |
Runs the next operation.
This simply runs the next operation
- Note
- You should not override this method
Implements ThreadOperation.
Definition at line 259 of file baseexperiment.cpp.
References OperationStatus::OperationEnded, and OperationStatus::OperationStarted.
|
virtual |
Saves the actual status of parameters into the ConfigurationParameters object passed.
- Parameters
-
params the configuration parameters object on which to save the actual parameters prefix the prefix to use to access the object configuration parameters
Implements Component.
Definition at line 209 of file baseexperiment.cpp.
References ConfigurationParameters::startObjectParameters().
void step | ( | ) |
Performs a single step for the current steppable operation.
The steppable operation must be paused, otherwise this function does nothing
- Note
- this function is thread-safe
Definition at line 294 of file baseexperiment.cpp.
|
virtual |
Forces the end of the experiment.
This is the implementation of the stop() function of ThreadOperation. If the opeation is sleeping, it is also resumed
- Note
- You should not override this method
Implements ThreadOperation.
Definition at line 275 of file baseexperiment.cpp.
|
virtualslot |
Forces the end of the current operation, if threaded.
This is a slot
- Parameters
-
wait if true waits for operation to actually stop, otherwise returns immediately
Definition at line 336 of file baseexperiment.cpp.
|
virtualslot |
Forces the end of the current operation, if threaded.
This is a slot. This calls stopCurrentOperation(false)
Definition at line 342 of file baseexperiment.cpp.
Referenced by BaseExperiment::BaseExperiment().
Friends And Related Function Documentation
|
friend |
The BaseExperimentFlowController class is friend to access our private members and functions.
Definition at line 361 of file baseexperiment.h.
|
friend |
The Notifee class is friend to access our private members and functions.
Definition at line 313 of file baseexperiment.h.
The documentation for this class was generated from the following files:
- experiments/include/baseexperiment.h
- experiments/src/baseexperiment.cpp