The class used to upload data. More...
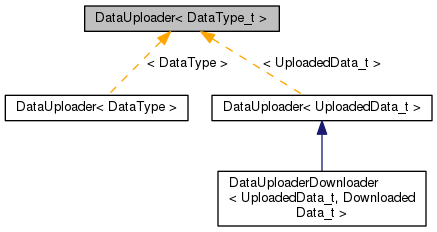
Public Types | |
typedef DataType_t | DataType |
The type of data being exchanged. More... | |
typedef farsa::DatumToUpload< DataType > | DatumToUpload |
A typedef to use the correct DatumToUpload. More... | |
enum | FullQueueBehavior { OverrideOlder, BlockUploader, IncreaseQueueSize, SignalUploader } |
The possible behaviors when the queue is full. More... | |
Public Member Functions | |
DataUploader (unsigned int queueSize, FullQueueBehavior b) | |
Constructor. More... | |
~DataUploader () | |
Destructor. More... | |
bool | associationBeforeUploadChecked () const |
Returns true if an exception is thrown if the user tries to upload a datum but no association is present. More... | |
void | checkAssociationBeforeUpload (bool v) |
Sets whether an exception has to be thrown if the user tries to upload a datum but no association is present. More... | |
DataType * | createDatum () |
Returns a pointer to an object that will be the next datum to upload. More... | |
bool | datumCreatedNotUploaded () const |
Returns true if a new datum has been created but not uploaded (i.e. createDatum() has been called but uploadDatum() hasn't) More... | |
bool | downloaderPresent () const |
Returns true if we are associated with a downloader. More... | |
unsigned int | getAvailableSpace () const |
Returns the number of data the queue can hold before becoming full. More... | |
FullQueueBehavior | getFullQueueBehavior () const |
Returns the FullQueueBehavior. More... | |
unsigned int | getNumDataInQueue () const |
Returns the number of data currently in the queue. More... | |
unsigned int | getQueueSize () const |
Returns the queue size. More... | |
void | uploadDatum () |
Adds the current datum to the queue. More... | |
Friends | |
class | GlobalUploaderDownloader |
GlobalUploaderDownloader is friend to access the queue. More... | |
Detailed Description
template<class DataType_t>
class farsa::DataUploader< DataType_t >
The class used to upload data.
See dataexchange.h for more information
- Warning
- Functions in this class are NOT thread safe
Definition at line 241 of file dataexchange.h.
Member Typedef Documentation
typedef DataType_t DataType |
The type of data being exchanged.
Definition at line 247 of file dataexchange.h.
typedef farsa::DatumToUpload<DataType> DatumToUpload |
A typedef to use the correct DatumToUpload.
Definition at line 252 of file dataexchange.h.
Member Enumeration Documentation
enum FullQueueBehavior |
The possible behaviors when the queue is full.
Definition at line 257 of file dataexchange.h.
Constructor & Destructor Documentation
DataUploader | ( | unsigned int | queueSize, |
FullQueueBehavior | b | ||
) |
Constructor.
- Parameters
-
queueSize the size of the queue. If the b parameter is IncreaseQueueSize, this is the initial size and the queue will never be shorter than this. The queue will always have at least one element (even if this is set to 0) b the behavior when the queue is full
Definition at line 1409 of file dataexchange.h.
~DataUploader | ( | ) |
Destructor.
Definition at line 1418 of file dataexchange.h.
Member Function Documentation
|
inline |
Returns true if an exception is thrown if the user tries to upload a datum but no association is present.
When this object is constructed, this is set to true
- Returns
- true if an exception is thrown if the user tries to upload a datum but no association is present
Definition at line 381 of file dataexchange.h.
|
inline |
Sets whether an exception has to be thrown if the user tries to upload a datum but no association is present.
When this object is constructed, this is set to true
- Parameters
-
v if true an exception is thrown when the user tries to upload a datum and no association is present
Definition at line 368 of file dataexchange.h.
DataType_t * createDatum | ( | ) |
Returns a pointer to an object that will be the next datum to upload.
Modify the object returned by this function, it will be the next datum to upload. If the queue is full, this function blocks if the FullQueueBehavior is set to BlockUploader, while it returns NULL if the FullQueueBehavior is set to SignalUploader
- Returns
- the object that will be the next datum
- Warning
- If you call this function remember to call uploadDatum() when done. A more convenient way to create data is to use DatumToUpload
Definition at line 1457 of file dataexchange.h.
References UploaderDownloaderAssociationNotPresentException::DownloaderNotPresent.
bool datumCreatedNotUploaded | ( | ) | const |
Returns true if a new datum has been created but not uploaded (i.e. createDatum() has been called but uploadDatum() hasn't)
- Returns
- true if a new datum has been created but not uploaded
Definition at line 1599 of file dataexchange.h.
bool downloaderPresent | ( | ) | const |
Returns true if we are associated with a downloader.
- Returns
- true if we are associated with a downloader
Definition at line 1429 of file dataexchange.h.
unsigned int getAvailableSpace | ( | ) | const |
Returns the number of data the queue can hold before becoming full.
If the FullQueueBehavior is IncreaseQueueSize, this function always returns at least 1
- Returns
- the number of data the queue can hold before becoming full
Definition at line 1437 of file dataexchange.h.
|
inline |
Returns the FullQueueBehavior.
- Returns
- the FullQueueBehavior
Definition at line 299 of file dataexchange.h.
unsigned int getNumDataInQueue | ( | ) | const |
Returns the number of data currently in the queue.
If the FullQueueBehavior is IncreaseQueueSize, this function can return a value greater than getQueueSize()
- Returns
- the number of data currently in the queue
Definition at line 1449 of file dataexchange.h.
|
inline |
void uploadDatum | ( | ) |
Adds the current datum to the queue.
If createDatum() hasn't been called, this function does nothing
Definition at line 1530 of file dataexchange.h.
Friends And Related Function Documentation
|
friend |
GlobalUploaderDownloader is friend to access the queue.
Definition at line 435 of file dataexchange.h.
The documentation for this class was generated from the following file:
- utilities/include/dataexchange.h