A class for bi-directional communication. More...
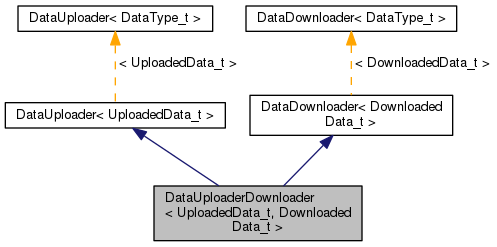
Public Types | |
typedef DownloadedData_t | DownloadedData |
The type of data being downloaded by this class. More... | |
typedef DataUploader< UploadedData >::FullQueueBehavior | FullQueueBehavior |
A typedef to easily access the FullQueueBehavior type. More... | |
typedef DataDownloader< DownloadedData >::NewDatumAvailableBehavior | NewDatumAvailableBehavior |
A typedef to easily access the NewDatumAvailableBehavior type. More... | |
typedef UploadedData_t | UploadedData |
The type of data being uploaded by this class. More... | |
![]() | |
typedef UploadedData_t | DataType |
The type of data being exchanged. More... | |
typedef farsa::DatumToUpload< DataType > | DatumToUpload |
A typedef to use the correct DatumToUpload. More... | |
enum | FullQueueBehavior |
The possible behaviors when the queue is full. More... | |
![]() | |
typedef DownloadedData_t | DataType |
The type of data being exchanged. More... | |
enum | NewDatumAvailableBehavior |
The possible behaviors when a new datum arrives. More... | |
Public Member Functions | |
DataUploaderDownloader (unsigned int uploadQueueSize, FullQueueBehavior fullQueueBehavior, NewDatumAvailableBehavior newDatumAvailableBehavior) | |
Constructor. More... | |
DataUploaderDownloader (unsigned int uploadQueueSize, FullQueueBehavior fullQueueBehavior, QObject *o) | |
Constructor. More... | |
DataUploaderDownloader (unsigned int uploadQueueSize, FullQueueBehavior fullQueueBehavior, NewDatumNotifiable< DownloadedData > *o) | |
Constructor. More... | |
~DataUploaderDownloader () | |
Destructor. More... | |
![]() | |
DataUploader (unsigned int queueSize, FullQueueBehavior b) | |
Constructor. More... | |
~DataUploader () | |
Destructor. More... | |
bool | associationBeforeUploadChecked () const |
Returns true if an exception is thrown if the user tries to upload a datum but no association is present. More... | |
void | checkAssociationBeforeUpload (bool v) |
Sets whether an exception has to be thrown if the user tries to upload a datum but no association is present. More... | |
DataType * | createDatum () |
Returns a pointer to an object that will be the next datum to upload. More... | |
bool | datumCreatedNotUploaded () const |
Returns true if a new datum has been created but not uploaded (i.e. createDatum() has been called but uploadDatum() hasn't) More... | |
bool | downloaderPresent () const |
Returns true if we are associated with a downloader. More... | |
unsigned int | getAvailableSpace () const |
Returns the number of data the queue can hold before becoming full. More... | |
FullQueueBehavior | getFullQueueBehavior () const |
Returns the FullQueueBehavior. More... | |
unsigned int | getNumDataInQueue () const |
Returns the number of data currently in the queue. More... | |
unsigned int | getQueueSize () const |
Returns the queue size. More... | |
void | uploadDatum () |
Adds the current datum to the queue. More... | |
![]() | |
DataDownloader (NewDatumAvailableBehavior b) | |
Constructor. More... | |
DataDownloader (QObject *o) | |
Constructor. More... | |
DataDownloader (NewDatumNotifiable< DataType > *o) | |
Constructor. More... | |
~DataDownloader () | |
Destructor. More... | |
const DataType * | downloadDatum () |
Returns a pointer to the next datum. More... | |
NewDatumAvailableBehavior | getNewDatumAvailableBehavior () const |
Returns the NewDatumAvailableBehavior. More... | |
unsigned int | getNumAvailableData () const |
Returns the number of available data. More... | |
bool | uploaderPresent () const |
Returns true if we are associated with an uploader. More... | |
Detailed Description
template<class UploadedData_t, class DownloadedData_t>
class farsa::DataUploaderDownloader< UploadedData_t, DownloadedData_t >
A class for bi-directional communication.
This class inherits from both DataUploader and DataDownloader. See dataexchange.h for more information
Definition at line 839 of file dataexchange.h.
Member Typedef Documentation
typedef DownloadedData_t DownloadedData |
The type of data being downloaded by this class.
Definition at line 850 of file dataexchange.h.
A typedef to easily access the FullQueueBehavior type.
Definition at line 855 of file dataexchange.h.
A typedef to easily access the NewDatumAvailableBehavior type.
Definition at line 860 of file dataexchange.h.
typedef UploadedData_t UploadedData |
The type of data being uploaded by this class.
Definition at line 845 of file dataexchange.h.
Constructor & Destructor Documentation
|
inline |
Constructor.
- Parameters
-
uploadQueueSize the size of the upload queue. If the b parameter is IncreaseQueueSize, this is the initial size and the queue will never be shorter than this. The queue will always have at least one element (even if this is set to 0) fullQueueBehavior the behavior of the uploader when the queue is full newDatumAvailableBehavior the behavior of the downloader when a new datum arrives. In this constructor this must be either NoNotification or NoNotificationBlocking, otherwise an exception is thrown
Definition at line 881 of file dataexchange.h.
|
inline |
Constructor.
This constructor sets the NewDatumAvailableBehavior of the downloader to QtEvent
- Parameters
-
uploadQueueSize the size of the upload queue. If the b parameter is IncreaseQueueSize, this is the initial size and the queue will never be shorter than this. The queue will always have at least one element (even if this is set to 0) fullQueueBehavior the behavior of the uploader when the queue is full o the object to send notifications to when a new datum is available. This must not be NULL.
Definition at line 903 of file dataexchange.h.
|
inline |
Constructor.
This constructor sets the NewDatumAvailableBehavior of the downloader to Callback
- Parameters
-
uploadQueueSize the size of the upload queue. If the b parameter is IncreaseQueueSize, this is the initial size and the queue will never be shorter than this. The queue will always have at least one element (even if this is set to 0) fullQueueBehavior the behavior of the uploader when the queue is full o the object whose callback has to be called when a new datum is available. This must not be NULL.
Definition at line 925 of file dataexchange.h.
|
inline |
Destructor.
Definition at line 934 of file dataexchange.h.
The documentation for this class was generated from the following file:
- utilities/include/dataexchange.h