The base class for robots that move on a plane. More...
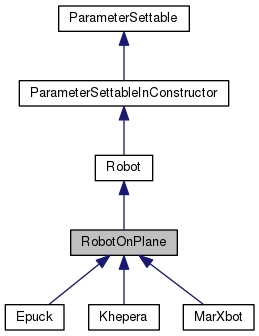
Public Member Functions | |
RobotOnPlane (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~RobotOnPlane () |
Destructor. More... | |
virtual bool | isKinematic () const =0 |
Returns true if the robot is in kinematic mode. More... | |
virtual real | orientation (const Box2DWrapper *plane) const =0 |
Returns the orientation of the robot. More... | |
virtual wVector | position () const =0 |
Returns the position of the robot. More... | |
virtual QColor | robotColor () const =0 |
Returns the color of the robot. More... | |
virtual real | robotHeight () const =0 |
Returns the height of the robot. More... | |
virtual real | robotRadius () const =0 |
Returns the radius of the robot. More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Saves the actual status of parameters into the ConfigurationParameters object passed. More... | |
virtual void | setOrientation (const Box2DWrapper *plane, real angle)=0 |
Sets the orientation of the robot. More... | |
void | setPosition (const Box2DWrapper *plane, const wVector &pos) |
Sets the position of the robot in the plane. More... | |
virtual void | setPosition (const Box2DWrapper *plane, real x, real y)=0 |
Sets the position of the robot in the plane. More... | |
![]() | |
Robot (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~Robot () |
Destructor. More... | |
![]() | |
ParameterSettableInConstructor (ConfigurationParameters &, QString) | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
virtual void | postConfigureInitialization () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
Static Public Member Functions | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Protected Member Functions | |
const QColor & | configuredRobotColor () const |
Returns the robot color specified by the configuration parameter. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
Detailed Description
The base class for robots that move on a plane.
This has some utility methods common to all robots that move on a plane. There are also protected static helper methods which can be used when re-implementing in subclasses. The only parameter of this class is the color of the robot (white by default)
Constructor & Destructor Documentation
RobotOnPlane | ( | ConfigurationParameters & | params, |
QString | prefix | ||
) |
Constructor.
- Parameters
-
params the configuration parameters object with parameters to use prefix the prefix to use to access the object configuration parameters. This is guaranteed to end with the separator character when called by the factory, so you don't need to add one
Definition at line 85 of file robots.cpp.
References ConfigurationParameters::getValue(), and ConfigurationHelper::throwUserConfigError().
|
virtual |
Destructor.
Definition at line 108 of file robots.cpp.
Member Function Documentation
|
inlineprotected |
Returns the robot color specified by the configuration parameter.
- Returns
- the robot color specified by the configuration parameter
Definition at line 250 of file robots.h.
Referenced by Epuck::Epuck(), Khepera::Khepera(), and MarXbot::MarXbot().
|
static |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups.
- Parameters
-
type is the name of the type regarding the description. The type is used when a subclass reuse the description of its parent calling the parent describe method passing the type of the subclass. In this way, the result of the method describe of the parent will be the addition of the description of the parameters of the parent class into the type of the subclass
Definition at line 100 of file robots.cpp.
References ParameterSettable::addTypeDescription(), and ParameterSettable::describe().
Referenced by MarXbot::describe(), Epuck::describe(), and Khepera::describe().
|
pure virtual |
|
pure virtual |
Returns the orientation of the robot.
This returns the angle around the z axis of the "plane". An angle of 0 means that the X axis of the plane and of the robot are coincident (positive angles follow the right-hand rule). This function returns the angle in radiants
- Parameters
-
plane the plane on which the robot is placed. You can use the plane returned by Arena::getPlane(), here.
- Warning
- the orientation returned by this function is not the same as the orientation set by setOrientation, there is a difference of (pi/2)
|
pure virtual |
|
pure virtual |
|
pure virtual |
Returns the height of the robot.
This is the height of the cylinder containing the robot. In some cases wheeled robots are modelled as cylinders to simplify calculations
- Returns
- the height of the robot
Implemented in Khepera, Epuck, and MarXbot.
Referenced by Arena::addRobots().
|
pure virtual |
Returns the radius of the robot.
This is the radius of the cylinder containing the robot. In some cases wheeled robots are modelled as cylinders to simplify calculations
- Returns
- the radius of the robot
Implemented in Khepera, Epuck, and MarXbot.
Referenced by Arena::addRobots().
|
virtual |
Saves the actual status of parameters into the ConfigurationParameters object passed.
This is not implemented, calling this causes an abort
- Parameters
-
params the configuration parameters object on which save actual parameters prefix the prefix to use to access the object configuration parameters.
Implements ParameterSettable.
Reimplemented in Khepera, Epuck, and MarXbot.
Definition at line 94 of file robots.cpp.
References Logger::error().
|
pure virtual |
Sets the orientation of the robot.
This modifies the angle around the z axis of the "plane". An angle of 0 means that the X axis of the plane and of the robot are coincident (positive angles follow the right-hand rule). This function wants the angle in radiants
- Parameters
-
plane the plane on which the robot is placed. You can use the plane returned by Arena::getPlane(), here. angle the new orientation in radiants
void setPosition | ( | const Box2DWrapper * | plane, |
const wVector & | pos | ||
) |
Sets the position of the robot in the plane.
The Z coordinate is discarded. The robot is placed on the face of the "plane" parallel to the local XY plane and with positive z coordinate. This method simply calls setPosition(real x, real y)
- Parameters
-
plane the plane on which the robot should be placed. You can use the plane returned by Arena::getPlane(), here. pos the new position (the z coordinate is discarded)
Definition at line 113 of file robots.cpp.
|
pure virtual |
Sets the position of the robot in the plane.
The robot is placed on the face of the "plane" parallel to the local XY plane and with positive z coordinate.
- Parameters
-
plane the plane on which the robot should be placed. You can use the plane returned by Arena::getPlane(), here. x the new x coordinate y the new y coordinate
The documentation for this class was generated from the following files:
- experiments/include/robots.h
- experiments/src/robots.cpp