An helper class for linear camera sensors of various robots. More...
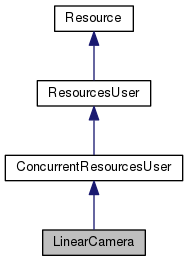
Public Member Functions | |
LinearCamera (WObject *obj, wMatrix mtr, double aperture, unsigned int numReceptors, double maxDistance, QColor backgroundColor) | |
Constructor. More... | |
LinearCamera (WObject *obj, wMatrix mtr, QVector< SimpleInterval > receptorsRanges, double maxDistance, QColor backgroundColor) | |
Constructor. More... | |
virtual | ~LinearCamera () |
Destructor. More... | |
const QColor & | colorForReceptor (int i) const |
Returns the color perceived by the i-th receptor. More... | |
void | drawCamera (bool d) |
Sets whether to draw the linear camera or not. More... | |
QColor | getBackgroundColor () const |
Returns the background color. More... | |
unsigned int | getNumReceptors () const |
Returns the number of receptors. More... | |
void | ignoreWalls (bool ignore) |
Sets whether walls are ignored or not. More... | |
void | setBackgroundColor (QColor color) |
Sets the background color. More... | |
void | update () |
Updates the sensor reading. More... | |
bool | wallsIgnored () const |
Whether walls are ignored or not. More... | |
![]() | |
void | addUsableResource (QString resource) |
void | addUsableResources (QStringList resources) |
void | declareResource (QString name, T *resource, QString lockBuddy="") |
void | deleteResource (QString name) |
T * | getResource (QString name, bool *resourceExists=NULL) |
bool | hasResource (QString name) const |
void | removeAllUsableResources () |
void | removeUsableResource (QString resource) |
void | removeUsableResources (QStringList resources) |
virtual void | shareResourcesWith (ResourcesUser *buddy) |
void | usableResources (QStringList resources) |
bool | usedResourcesExist (QStringList *nonExistingResources=NULL) const |
Additional Inherited Members | |
![]() | |
typedef Resource::ResourceChangeType | ResourceChangeType |
![]() | |
enum | ResourceChangeType |
![]() | |
T * | getResource () |
![]() | |
ResourcesUser (const ResourcesUser &other) | |
virtual void | notifyResourceChange (ResourceHandler *resource, ResourceChangeType changeType)=0 |
ResourcesUser & | operator= (const ResourcesUser &other) |
![]() | |
ResourceCollectionHolder | m_resources |
Detailed Description
An helper class for linear camera sensors of various robots.
This class has all that is needed to implement a linear camera sensor. It is fully parametrized, so to implement an actual sensor one just needs to instantiate it with the correct set of parameters. The camera is attached to an object and its transformation matrix is relative to the object to which it is attached. The up vector is the local Z axis, the camera points towards X and is on the XY plane. The camera only takes into account the objects in the "2dobjects" resource (a list of PhyObject2DWrapper objects). The number of receptors, their position and their aperture can be specified in two ways. The easiest one is to set the aperture and the number of receptors: all receptors will have the same range and will be placed symmetrically with respect to the X axis. The more general way is to supply a list of SimpleIntervals, where each interval represents a single receptor. This way you can have receptors of different sizes, overlapping receptors, blind spots and receptors with a custom order (the order of receptors is the same as in the list of intervals). Moreover the sensor takes into account visual occlusion. To work, this class need to access the arena object, so a resource named "arena" which points to the instance of the arena should be present.
- Note
- The current implementation of the camera doesn't work well with occluding objects. In fact the occlusion is only computed on the basis of the distance between the object center and the camera. If however you have e.g. a cylinder in front of big wall, the cylinder can be more distant than the wall and so being considered behind the wall. For this reason it is possible to ignore all walls using the ignoreWalls() function (this is usefult if e.g. you have only perimetral walls of the same color as the background)
- When more than one object of different colors hits a single receptor, here we take the average of the colors (weighted by the percentage of the receptor occupied by each object). This is not physically correct, so please be careful when using big receptors with a lot of objects of different colors.
Constructor & Destructor Documentation
LinearCamera | ( | WObject * | obj, |
wMatrix | mtr, | ||
double | aperture, | ||
unsigned int | numReceptors, | ||
double | maxDistance, | ||
QColor | backgroundColor | ||
) |
Constructor.
Use this constructor to have receptors that have the same aperture and are symmetrical with respect to the X axis
- Parameters
-
obj the object to which the sensor is attached. This cannot be NULL mtr the transformation matrix relative to the object to which the sensor is attached. The up vector is the local Z axis, the camera points towards X and is on the XY plane. aperture the aperture of the sensor in radiants numReceptors the number of receptors maxDistance the distance above which objects are not seen anymore backgroundColor the background color (used when no object is perceived by a given receptor)
Definition at line 1005 of file sensors.cpp.
References ConcurrentResourcesUser::addUsableResource().
LinearCamera | ( | WObject * | obj, |
wMatrix | mtr, | ||
QVector< SimpleInterval > | receptorsRanges, | ||
double | maxDistance, | ||
QColor | backgroundColor | ||
) |
Constructor.
Use this contructor to be able to directly specify the list of receptors
- Parameters
-
obj the object to which the sensor is attached. This cannot be NULL mtr the transformation matrix relative to the object to which the sensor is attached. The up vector is the local Z axis, the camera points towards X and is on the XY plane. receptorsRanges the list of ranges for receptors. Angles are in radiants maxDistance the distance above which objects are not seen anymore backgroundColor the background color (used when no object is perceived by a given receptor)
Definition at line 1023 of file sensors.cpp.
References ConcurrentResourcesUser::addUsableResource().
|
virtual |
Destructor.
Definition at line 1041 of file sensors.cpp.
Member Function Documentation
|
inline |
Returns the color perceived by the i-th receptor.
- Parameters
-
i the index of the photoreceptor to return
- Returns
- the color perceived by the receptor
Definition at line 544 of file sensors.h.
Referenced by MarXbotLinearCameraSensorNew::update().
void drawCamera | ( | bool | d | ) |
Sets whether to draw the linear camera or not.
- Parameters
-
d if true the camera is drawn, if false it is not
Definition at line 1237 of file sensors.cpp.
|
inline |
|
inline |
Returns the number of receptors.
- Returns
- the number of receptors
Definition at line 554 of file sensors.h.
Referenced by LinearCamera::update().
|
inline |
|
inline |
void update | ( | ) |
Updates the sensor reading.
Definition at line 1109 of file sensors.cpp.
References LinearCamera::getNumReceptors(), Arena::getObjects(), WObject::matrix(), farsa::max(), farsa::min(), farsa::normalizeRad(), and LinearCameraGraphic::setPerceivedColors().
Referenced by MarXbotLinearCameraSensorNew::update().
|
inline |
The documentation for this class was generated from the following files:
- experiments/include/sensors.h
- experiments/src/sensors.cpp