Genetic algorithm from evorobot more or less (spare parts) More...
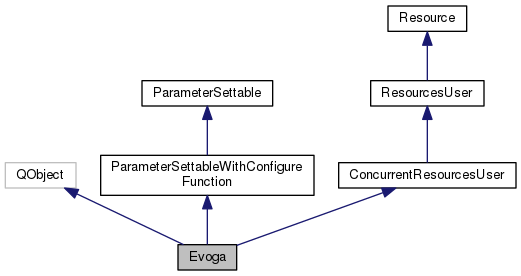
Classes | |
class | Population |
A class modelling a population of genomes. More... | |
Public Slots | |
void | doNextStep () |
Go ahead of one step if the EvoGa is in step-by-step modality, otherwise do nothing. More... | |
void | enableStepByStep (bool enable) |
enable/disable the step-by-step modality of evolution process More... | |
Signals | |
void | endGeneration (int generation, double fmax, double faverage, double fmin) |
emitted when a generation is ended More... | |
void | finished () |
emitted when the evolution has been finished or stopped More... | |
void | recoveredInterruptedEvolution (QString statfile) |
emitted when an interrupted evolution has been recovered and it's going to start from there More... | |
void | startingReplication (int replication) |
emitted when a replication is going to start More... | |
Public Member Functions | |
Evoga () | |
Constructor. More... | |
~Evoga () | |
Destructor. More... | |
virtual QString | bestsFilename (unsigned int seed) |
Returns the name of the file with the best genomes for the given seed. More... | |
virtual QString | bestsFilename () |
Returns the template name (regular expression) for "best genomes" files. More... | |
bool | commitStep () |
this method marks the point on which it is called as a commited step of evolution More... | |
void | computeFStat () |
Calculate the average, minimal and maximal fitness of the current population Assume that the fitness is always positive. More... | |
void | computeFStat2 () |
Calculate the average, minimal and maximal fitness of the current population Assume that the fitness can also be negative. More... | |
virtual void | configure (ConfigurationParameters ¶ms, QString prefix) |
Configures the object using a ConfigurationParameters object. More... | |
void | copyGenes (int from, int to, int mut) |
Copy the genotype of an individual into the genotype of a second individual and introduce mutations This function is used by the steadystate genetic algorithm to store the child in the genome matrix at position population+1 and eventually to use the genome of the child to overwrite the genome of the worst individual or discarded. More... | |
virtual void | doNotUseMultipleThreads () |
Forces execution of the GA using no threads (i.e., in the current thread) More... | |
double | drand () |
return a random floating point value generated with an uniform distribution in the range[0.0, 1.0] More... | |
virtual void | evolveAllReplicas () |
Evolves all replications of the ga process. More... | |
void | evolveGenerational () |
Main function of the Genetic Algorithm (Generational, Truncation Selection Version) The size of the population is set equal to nreproducing*noffspring Each individual is allowed to generate a variable number of offspring (noffspring parameter) Every generation the best "nreproducing" individuals are allowed to reproduce At reproduction the entire population is replaced by offspring unless the elitism parameter is greated than 0 When elitism is used, each individual is allowed to produce one or more offspring without mutations (i.e. More... | |
void | evolveSpecializerSteadyState () |
Main function of the Genetic Algorithm developed by Jonata. More... | |
void | evolveSpecializerSteadyState2 () |
Main function of the Genetic Algorithm developed by Jonata - Team Version. More... | |
void | evolveSteadyState () |
Main function of the Genetic Algorithm (Steady State Version) Each individual of the population is allowed to produce an offspring that is used to replace the current worst individual or discarded depending on whether its fitness is better or not of the worst individuals During each generation individuals are re-evaluated to avoid that individuals that happened to be luck in their first evaluation continue to be overestimated with respect to their real capacities The mutation rate is initially set to 50% and then progressively reduced of 1% each generation until it reach the value specified in the mutation rate parameter This initially hight mutation rate is used to explore many random solutions during the initial generations to avoid premature convergence The size of the population is set equal to nreproducing*noffspring however, the number of offsrping is always 1 (it is independent from the noffpring parameter) More... | |
virtual QString | generationFilename (unsigned int generation, unsigned int seed) |
Returns the name of the file containing the given generation for the given seed. More... | |
virtual QString | generationFilename () |
Returns the template name (regular expression) for "generation" files. More... | |
int * | getBestGenes (int ind) |
Return a pointer to the genome of one individual stored in the bestgenome matrix. More... | |
virtual unsigned int | getCurrentGeneration () |
Returns the current generation. More... | |
virtual double | getCurrentMutationRate () |
Returns the current mutation rate. More... | |
virtual unsigned int | getCurrentSeed () |
Returns the current seed. More... | |
QString | getEvolutionType () |
virtual EvoRobotExperiment * | getEvoRobotExperiment () |
Returns a pointer to the EvoRobotExperiment object. More... | |
virtual QVector< EvoRobotExperiment * > | getEvoRobotExperimentPool () |
Returns a list of pointers to the EvoRobotExperiment objects used by the ga to evaluate agents. More... | |
int * | getGenes (int ind) |
Return a pointer to the genome of one individual of the current population. More... | |
virtual int * | getGenesForIndividual (unsigned int id) |
Returns the array of genes for the given individual. More... | |
void | getGenome (int frombestgenome, int togenome, int mut) |
Copy a genome stored in the bestgenome matrix into the genome of the current population Apply mutation during the copy. More... | |
void | getLastFStat (double &min, double &max, double &average) |
return the last value of min, max and average fitness More... | |
double | getNoise (double minn, double maxn) |
Unused function (to be removed) More... | |
virtual unsigned int | getNumOfGenerations () |
Returns the number of generations to do. More... | |
virtual unsigned int | getNumReplications () |
Returns the number of replications. More... | |
void | getPheParametersAndMutationsFromEvonet () |
transfer .phe info inside GA More... | |
virtual unsigned int | getStartingSeed () |
Returns the starting seed. More... | |
bool | isEnabledStepByStep () |
return true is the step-by-step modality is enabled, false otherwise More... | |
bool | isStopped () |
return true if the evolution process has been stopped with stop() More... | |
int | loadallg (int gen, const char *filew) |
Load the genome of the population from a file. More... | |
void | loadallTeams (int gen, const char *filew, QVector< QVector< int > > &teams) |
Load the teams of the population from a file. More... | |
void | loadgenotype (FILE *fp, int ind) |
Load the genome of one individual in a text file. More... | |
virtual unsigned int | loadGenotypes (QString filename) |
Loads genotypes from the given file. More... | |
int | loadStatistics (char *filename) |
Load the fitness statistics from a .fit file Return the number of loaded individuals (0 if the file does not exists) More... | |
int | mrand (int i) |
return a random integer generated with an uniform distribution in the range [0, i-1] More... | |
void | mreproduce () |
Identify the best individuals and copy their genotypes into the bestgenome Matrix It also save the best genotypes in the Bx.gen files Assume that the best individuals have the lowest fitness (minimization) This function is not used and it is to be removed. More... | |
int | mutate (int w, double mut) |
Mutate one gene Since genes are store as integer, this function first trasform them into 8 bits and then mutate (i.e. More... | |
virtual unsigned int | numLoadedGenotypes () const |
Returns number of loaded genotypes. More... | |
virtual void | postConfigureInitialization () |
This function is called after all linked objects have been configured. More... | |
void | printBest () |
Print the fitness of the best individuals of the current population. More... | |
void | printPop () |
Print the fitness of the individuals of the current population. More... | |
void | putGenome (int fromgenome, int tobestgenome) |
Copy the genome of one individual to the bestgenome matrix in a specific position. More... | |
void | randomizePop () |
Randomize the genome of the population. More... | |
void | reproduce () |
Identify the best individuals and copy their genotypes into the bestgenome Matrix It also save the best genotypes in the Bx.gen files. More... | |
void | resetGenerationCounter () |
Reset the generation counter to 0. More... | |
void | resetStop () |
reset the stopped status to false More... | |
virtual QString | retentionsFilename (unsigned int seed) |
Returns the name of the file with statistics (retentions) for the given seed. More... | |
int | rouletteWheel (QVector< double > candidates) |
perform a roulette wheel and return the winner's index More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Save the actual status of parameters into the ConfigurationParameters object passed. More... | |
void | saveagenotype (FILE *fp, int ind) |
Save the genome of one individual in a text file. More... | |
void | saveallg () |
Save the genome of the current population in a G?S?.gen file. More... | |
void | saveallgComposed (QVector< QVector< int > > composedGen) |
Save the composed genome of the current population in a G?S?.composed.gen file. More... | |
void | saveBestFitness () |
void | saveBestInd () |
Append the genome of the best individual of the current population to the BdSd.gen file. More... | |
void | saveBestTeam (QVector< QVector< int > > teams, QVector< double > fitness) |
Append the best team of the current population to the BdSd.composed.gen file. More... | |
void | saveFStat () |
Save the average, minimal and maximal fitness by appending a line to the statSd.fit file. More... | |
void | saveRStat (QVector< int > subsVec) |
Save the information regarding the offspring retention by appending a line to the retention statistics file (function retentions. More... | |
void | saveStatistics (int popSize, int rp) |
virtual void | setCurrentMutationRate (double mutation_rate) |
Set the current mutation rate to use. More... | |
void | setInitialPopulation (int *) |
Overwrite the genome of all individuals with a manually specify vector of parameters (loaded from a .phe file) This enable to manually fix some of the parameters The Evonet::DEFAULT_VALUE value is used to indicate the parameters are not fixed and that are replaced with randomly generated value. More... | |
void | setMutations (float *) |
Set the mutation rate of each parameter. More... | |
void | setSeed (int s) |
Initialize the random number generator. More... | |
virtual QString | statisticsFilename (unsigned int seed) |
Returns the name of the file with statistics (fitness) for the given seed. More... | |
void | stop () |
stop the running evolution process as soon as possible More... | |
void | updateGenomeFromEvonet (int ind) |
update the genome of the individual to match the current weights of Evonet More... | |
void | xnes () |
Main function of the xNES Algorithm (exponential Natural Evolution Strategies) The size of the population is set to 1 (i.e. More... | |
void | xnesComputeStats () |
float * | xnesGetGenes (int ind) |
float * | xnesGetGenesForIndividual (unsigned int id) |
int | xnesLoadAllGen (int gen, const char *filew) |
void | xnesLoadGen (FILE *fp, int ind) |
void | xnesSaveAllGen () |
void | xnesSaveBest (int bi) |
void | xnesSaveBestPhe (int gen, int bi) |
void | xnesSaveGen (FILE *fp, float *genotype) |
void | xnesSaveStats () |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
![]() | |
void | addUsableResource (QString resource) |
void | addUsableResources (QStringList resources) |
void | declareResource (QString name, T *resource, QString lockBuddy="") |
void | deleteResource (QString name) |
T * | getResource (QString name, bool *resourceExists=NULL) |
bool | hasResource (QString name) const |
void | removeAllUsableResources () |
void | removeUsableResource (QString resource) |
void | removeUsableResources (QStringList resources) |
virtual void | shareResourcesWith (ResourcesUser *buddy) |
void | usableResources (QStringList resources) |
bool | usedResourcesExist (QStringList *nonExistingResources=NULL) const |
Static Public Member Functions | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Public Attributes | |
int | numModules |
The number of modules that a genotype should use. More... | |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
Static Public Attributes | |
static const int | MAXINDIVIDUALS = 1000 |
constant defining the maximimum number of individuals that Evoga can manage More... | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
Protected Attributes | |
bool | averageIndividualFitnessOverGenerations |
Whether to average an individual fitness with its previous one or not. More... | |
bool | backPropOffspringFitnessIncrease |
Flag whether to increase the fitness of the backpropagation offspring. More... | |
float | backPropOffspringFitnessIncreasePercentage |
Percentage of increase of the fitness of the backpropagation offspring. More... | |
int | backPropOffspringUtilityRank |
The utility rank of the backpropagation offspring in the second type of combination with xNES. More... | |
Population | bestgenome |
The matrix that contains a copy of the genome of the best individuals. More... | |
int | ccycle |
The current cycle. More... | |
int | cgen |
current generation More... | |
double | crossRate |
The probability that a crossover will occur in an offspring team – specializerSteadyState. More... | |
int | currentSeed |
The seed of the current generation (used for example to generate the name of the G?S?.gen files) More... | |
bool | debugPrintInfoToFile |
Flags whether to print debug information about the xNES algorithm to file. More... | |
bool | dissociateCovMatrix |
Flags whether to dissociate back-propagation effect on the xNES covariance matrix update. More... | |
bool | dissociateInd |
Flags whether to dissociate back-propagation effect on the xNES individual update. More... | |
bool | elitism |
if the Evoga will use elitism or not More... | |
QString | evolutionType |
specify the type of the evolution process to execute This string can only assume two values at the moment: 'steadyState' or 'generational' or 'xnes' More... | |
EvoRobotExperiment * | exp |
the EvoRobotExperiment More... | |
double | faverage |
double | fbest |
fitness best More... | |
int | fbestgen |
fitness best generation (maximum) More... | |
double | fmax |
double | fmin |
fitness statistics (minimum, maximum, and average fitness) More... | |
double | gaussianMean |
Mean of the gaussian distribution. More... | |
double | gaussianStdDev |
Standard deviation of the gaussian distribution. More... | |
Population | genome |
The matrix that contain the genome of the corrent population. More... | |
QVector< float * > | genotypes |
int | glen |
genome lenght (number of free parameters) More... | |
double | initial_mutation |
The initial mutation rate setted when steadyState algorithm is used. More... | |
bool | isStepByStep |
if step-by-step modality is enabled or not More... | |
double | limitationFactor |
The limitation factor that will multiply the offsprings' fitness. More... | |
bool | limitRetention |
Wheather the retention should be limited or not. More... | |
int | loadedIndividuals |
The number of individual genome loaded from a .gen file into the genome matrix (-1 when none has been loaded) More... | |
bool | minimization |
Flags whether the fitness function is set to solve a minimization problem. More... | |
double | modulesMutationRate |
The probability that a module will be mutated on a team – specializerSteadyState. More... | |
bool | mutateOnlyRelatives |
Wheater a module should be replace by its own offspring or by any offspring – specializerSteadyState. More... | |
double | mutation |
The mutation rate can vary from 0 to 1 continuosly to express probability in the range [0, 100%]. More... | |
double | mutationdecay |
The amount of reduction of the mutation generations during the first generations until it reaches the specified mutation rate. More... | |
double | mutationLearningRate |
The mutation learning rate used to generate offspring from backprop array. More... | |
double | mutationRange |
The mutation range used in XNES algorithm to initialize the individual. More... | |
float * | mutations |
vector of parameter-specific mutations More... | |
QMutex | mutexStepByStep |
the mutex used for controlling the step-by-step modality More... | |
int | noffspring |
number of sons More... | |
int | nogenerations |
The number of generations specified with the ngenerations parameter. More... | |
int | nreplications |
The number of replications specified with the corresponding parameter. More... | |
int | nreproducing |
number of individuals allowed to reproduce More... | |
double * | ntfitness |
A pointer to a vector used to store the total number of trials in which an unchanged individuals have been evaluated. More... | |
int | numStartInd |
Number of possible individuals from which finding out the starting individual of XNES. More... | |
unsigned int | numThreads |
The number of concurrent threads to use. More... | |
double | offspringMutRange |
The mutation range used in XNES algorithm to generate the offspring. More... | |
double | offspringStdDev |
Standard deviation to generate offspring. More... | |
double | overwriteRate |
The probability that a module will be overwritten by another one – specializerSteadyState. More... | |
int | pheGen |
How often (in terms of number of generations) the best phenotype must be saved. More... | |
int | popSize |
population size More... | |
double | rankBasedProb |
The probability that the individuals with the highest fitness will be selected. More... | |
int | savebest |
save best savebest parents by default equals 1 More... | |
ConfigurationParameters | savedConfigurationParameters |
A copy of the ConfigurationParameters object that was passed to our configure method. More... | |
QString | savedExperimentPrefix |
A copy of the prefix for experiment parameters. More... | |
int | savePopulationEachNGenerations |
How often (i.e. how many generations) we want to same the population genome in a .gen file. More... | |
bool | saveRetStat |
Whether to save retentions statistics or not. More... | |
int | seed |
The number used to initialized the seed of the first replication (successive replication use incremented numbers) More... | |
QString | selectionType |
The type of selection that will be used to define which individuals will survive. More... | |
bool | specialUtilityRanking |
Flags whether to use a special version to compute utility ranking. More... | |
bool | standardNes |
Flags whether the standard xNES algorithm must be used (i.e. the parameters are set according to the paper) More... | |
double ** | statfit |
A matrix that store the statistics (average, min, and max fitness) for all generations. More... | |
bool | stopEvolution |
Flag used to request to end the evolutionary process. More... | |
double | targetRetentionRate |
The target retention rate. More... | |
double * | terror |
A pointer to a vector used to store the sum of the errors obtained during different evaluations of an unmodified individual. More... | |
double * | tfitness |
A pointer to a vector used to store the sum of the fitness obtained during different evaluations of an unmodified individual. More... | |
bool | useGaussian |
Flag to decide whether using gaussian distribution to generate offspring. More... | |
bool | variableStdDev |
Flags whether the standard deviation to generate offspring is constant or not. More... | |
QWaitCondition | waitForNextStep |
the wait condition for waiting on step-by-step modality More... | |
bool | xnesCombination |
Flag to decide whether combining xnes with backpropagation algorithm. More... | |
int | xnesCombinationType |
The type of combination between xnes and backpropagation algorithms. More... | |
![]() | |
ResourceCollectionHolder | m_resources |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
typedef Resource::ResourceChangeType | ResourceChangeType |
![]() | |
enum | ResourceChangeType |
![]() | |
void | notifyChangesToParam (QString paramName) |
![]() | |
T * | getResource () |
virtual void | resourceChanged (QString name, ResourceChangeType changeType) |
![]() | |
ResourcesUser (const ResourcesUser &other) | |
virtual void | notifyResourceChange (ResourceHandler *resource, ResourceChangeType changeType)=0 |
ResourcesUser & | operator= (const ResourcesUser &other) |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
Detailed Description
Genetic algorithm from evorobot more or less (spare parts)
Mandatory Resources that the EvoRobotExperiment has to declare:
Optional Resources that the EvoRobotExperiment may declare:
- "evonet": a pointer to the EvoNet
Constructor & Destructor Documentation
~Evoga | ( | ) |
Destructor.
Definition at line 360 of file evoga.cpp.
References Evoga::exp, Evoga::mutations, Evoga::nogenerations, Evoga::ntfitness, Evoga::statfit, Evoga::terror, and Evoga::tfitness.
Member Function Documentation
|
virtual |
|
virtual |
bool commitStep | ( | ) |
this method marks the point on which it is called as a commited step of evolution
This method allow to divide the code on blocks where each of it can be considered as a single step of the evolution process. These key points define the behaviour when the advancement step-by-step has been requested. When the advancement of the evolution is on "step-by-step" (enableStepByStep method), everytime this method is encountered the process will stop waiting to pass over when the user send a request to perform an another step.
This method handle also the stopping process of the evolution. In fact, it return true if the evolution process has been stopped with stop() This function return true only between the calls to stop() and to resetStop() methods:
stop(); commitStep(); // <– it will return true resetStop(); commitStep(); // <– it will return false
- Note
- resetStop() is not called automatically by the Evoga after the exit for a stop, but it's called by the EvoRobotComponent after the execution of action 'stop'; If you want to use the Evoga in your custom Component remember to call resetStop()
Definition at line 3040 of file evoga.cpp.
References Evoga::isStepByStep, Evoga::mutexStepByStep, Evoga::stopEvolution, and Evoga::waitForNextStep.
Referenced by EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
void computeFStat | ( | ) |
Calculate the average, minimal and maximal fitness of the current population Assume that the fitness is always positive.
Definition at line 706 of file evoga.cpp.
References Evoga::cgen, Evoga::fbest, Evoga::fbestgen, Evoga::fmin, farsa::max(), farsa::min(), Evoga::popSize, Evoga::statfit, and Evoga::tfitness.
Referenced by Evoga::mreproduce(), and Evoga::reproduce().
void computeFStat2 | ( | ) |
Calculate the average, minimal and maximal fitness of the current population Assume that the fitness can also be negative.
Definition at line 732 of file evoga.cpp.
References Evoga::cgen, Evoga::fbest, Evoga::fbestgen, Evoga::fmin, farsa::max(), farsa::min(), Evoga::ntfitness, Evoga::popSize, Evoga::statfit, and Evoga::tfitness.
Referenced by Evoga::evolveSteadyState().
|
virtual |
Configures the object using a ConfigurationParameters object.
- Parameters
-
params the configuration parameters object with parameters to use prefix the prefix to use to access the object configuration parameters. This is guaranteed to end with the separator character when called by the factory, so you don't need to add one
Implements ParameterSettableWithConfigureFunction.
Definition at line 2784 of file evoga.cpp.
References Evoga::averageIndividualFitnessOverGenerations, Evoga::backPropOffspringFitnessIncrease, Evoga::backPropOffspringFitnessIncreasePercentage, Evoga::backPropOffspringUtilityRank, Evoga::bestgenome, Evoga::crossRate, Evoga::debugPrintInfoToFile, Evoga::dissociateCovMatrix, Evoga::dissociateInd, Evoga::elitism, Logger::error(), Evoga::evolutionType, Evoga::exp, Evoga::gaussianMean, Evoga::gaussianStdDev, Evoga::genome, ConfigurationHelper::getBool(), ConfigurationHelper::getDouble(), ConfigurationHelper::getInt(), ConfigurationParameters::getObjectFromGroup(), ConfigurationHelper::getString(), ConfigurationParameters::getValue(), Logger::info(), Evoga::initial_mutation, Evoga::limitRetention, Evoga::minimization, Evoga::modulesMutationRate, Evoga::mutateOnlyRelatives, Evoga::mutation, Evoga::mutationdecay, Evoga::mutationLearningRate, Evoga::mutationRange, Evoga::noffspring, Evoga::nogenerations, Evoga::nreplications, Evoga::nreproducing, Evoga::numModules, Evoga::numStartInd, Evoga::numThreads, Evoga::offspringMutRange, Evoga::offspringStdDev, Evoga::overwriteRate, Evoga::pheGen, Evoga::rankBasedProb, Evoga::savebest, Evoga::savedConfigurationParameters, Evoga::savedExperimentPrefix, Evoga::savePopulationEachNGenerations, Evoga::saveRetStat, Evoga::seed, Evoga::selectionType, EvoRobotExperiment::setEvoga(), Evoga::specialUtilityRanking, Evoga::standardNes, Evoga::targetRetentionRate, Evoga::useGaussian, Evoga::variableStdDev, Evoga::xnesCombination, and Evoga::xnesCombinationType.
void copyGenes | ( | int | from, |
int | to, | ||
int | mut | ||
) |
Copy the genotype of an individual into the genotype of a second individual and introduce mutations This function is used by the steadystate genetic algorithm to store the child in the genome matrix at position population+1 and eventually to use the genome of the child to overwrite the genome of the worst individual or discarded.
- Parameters
-
from The id of the individual genome to reproduce to The id of the location where the reproduced individual is stored mut A binary flag that specify whether mutation should be introduced of not
Definition at line 455 of file evoga.cpp.
References Evonet::DEFAULT_VALUE, Evoga::genome, Evoga::glen, Evoga::mutate(), Evoga::mutation, and Evoga::mutations.
Referenced by Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
static |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups.
- Parameters
-
type is the name of the type regarding the description. The type is used when a subclass reuse the description of its parent calling the parent describe method passing the type of the subclass. In this way, the result of the method describe of the parent will be the addition of the description of the parameters of the parent class into the type of the subclass
Definition at line 2886 of file evoga.cpp.
References ParameterSettable::addTypeDescription(), ParameterSettable::IsMandatory, and ParameterSettable::MaxInteger.
|
slot |
Go ahead of one step if the EvoGa is in step-by-step modality, otherwise do nothing.
Definition at line 3070 of file evoga.cpp.
References Evoga::waitForNextStep.
|
virtual |
Forces execution of the GA using no threads (i.e., in the current thread)
Definition at line 3170 of file evoga.cpp.
References Evoga::numThreads.
Referenced by EvoRobotExperiment::postConfigureInitialization().
double drand | ( | ) |
return a random floating point value generated with an uniform distribution in the range[0.0, 1.0]
- Parameters
-
i The maximum value of the integer to be generated
Definition at line 383 of file evoga.cpp.
Referenced by Evoga::getNoise(), and Evoga::mutate().
|
slot |
enable/disable the step-by-step modality of evolution process
When the step-by-step modality is enabled, the evolution process will block in some specific points waiting the user command to go ahead
- Parameters
-
enable if true enable the step-by-step, if false disable it
Definition at line 3058 of file evoga.cpp.
References Evoga::isStepByStep, and Evoga::waitForNextStep.
|
signal |
emitted when a generation is ended
- Parameters
-
generation is the number of generation finished fmax is the maximum fitness value faverage is the average fitness fmin is the minimum fitness value
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
virtual |
Evolves all replications of the ga process.
Definition at line 3016 of file evoga.cpp.
References Logger::error(), Evoga::evolutionType, Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::numModules, Evoga::stopEvolution, and Evoga::xnes().
Referenced by EvoRobotComponent::evolve(), and EvolveOperation::run().
void evolveGenerational | ( | ) |
Main function of the Genetic Algorithm (Generational, Truncation Selection Version) The size of the population is set equal to nreproducing*noffspring Each individual is allowed to generate a variable number of offspring (noffspring parameter) Every generation the best "nreproducing" individuals are allowed to reproduce At reproduction the entire population is replaced by offspring unless the elitism parameter is greated than 0 When elitism is used, each individual is allowed to produce one or more offspring without mutations (i.e.
identical copies)
Definition at line 2676 of file evoga.cpp.
References Evoga::commitStep(), EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::endGeneration(), EvoRobotExperiment::endGeneration(), Evoga::exp, Evoga::fbest, Evoga::fmin, Evoga::genome, Evoga::getCurrentSeed(), EvoRobotExperiment::getFitness(), Evoga::getGenes(), Evoga::getPheParametersAndMutationsFromEvonet(), Evoga::getStartingSeed(), ConcurrentResourcesUser::hasResource(), Logger::info(), EvoRobotExperiment::initGeneration(), Evoga::loadallg(), EvoRobotExperiment::newGASeed(), Evoga::nogenerations, Evoga::nreplications, Evoga::popSize, Evoga::randomizePop(), Evoga::recoveredInterruptedEvolution(), Evoga::reproduce(), Evoga::resetGenerationCounter(), Evoga::saveallg(), Evoga::savePopulationEachNGenerations, EvoRobotExperiment::setNetParameters(), Evoga::setSeed(), Evoga::startingReplication(), and Evoga::tfitness.
Referenced by Evoga::evolveAllReplicas().
void evolveSpecializerSteadyState | ( | ) |
Main function of the Genetic Algorithm developed by Jonata.
Definition at line 3187 of file evoga.cpp.
References Evoga::cgen, Evoga::commitStep(), Evoga::copyGenes(), Evoga::currentSeed, EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::endGeneration(), EvoRobotExperiment::endGeneration(), Evoga::exp, Evoga::fmin, Evoga::genome, EvoRobotExperiment::getFitness(), Evoga::getGenesForIndividual(), ConfigurationParameters::getObjectFromGroup(), Evoga::getPheParametersAndMutationsFromEvonet(), Evoga::getStartingSeed(), ConcurrentResourcesUser::hasResource(), Logger::info(), EvoRobotExperiment::initGeneration(), Evoga::initial_mutation, Evoga::isStopped(), Evoga::limitationFactor, Evoga::limitRetention, Evoga::loadallg(), Evoga::loadedIndividuals, EvoRobotExperiment::m_weightIndividual, Evoga::mutation, Evoga::mutationdecay, Evoga::nogenerations, Evoga::nreplications, Evoga::numThreads, Evoga::popSize, Evoga::randomizePop(), Evoga::recoveredInterruptedEvolution(), Evoga::resetGenerationCounter(), Evoga::saveallg(), Evoga::savedConfigurationParameters, Evoga::savedExperimentPrefix, Evoga::saveFStat(), Evoga::savePopulationEachNGenerations, Evoga::saveRetStat, Evoga::saveRStat(), EvoRobotExperiment::setEvoga(), Evoga::setSeed(), Evoga::startingReplication(), Evoga::statfit, Evoga::targetRetentionRate, and Logger::warning().
Referenced by Evoga::evolveAllReplicas().
void evolveSpecializerSteadyState2 | ( | ) |
Main function of the Genetic Algorithm developed by Jonata - Team Version.
Definition at line 3405 of file evoga.cpp.
References Evoga::cgen, Evoga::commitStep(), Evoga::copyGenes(), Evoga::crossRate, Evoga::currentSeed, EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::endGeneration(), EvoRobotExperiment::endGeneration(), Evoga::exp, Evoga::fmin, Evoga::genome, EvoRobotExperiment::getFitness(), Evoga::getGenesForIndividual(), ConfigurationParameters::getObjectFromGroup(), Evoga::getPheParametersAndMutationsFromEvonet(), Evoga::getStartingSeed(), ConcurrentResourcesUser::hasResource(), FitnessAndId::id, Logger::info(), EvoRobotExperiment::initGeneration(), Evoga::initial_mutation, Evoga::limitationFactor, Evoga::limitRetention, Evoga::loadallg(), Evoga::loadallTeams(), Evoga::loadedIndividuals, EvoRobotExperiment::m_weightIndividual, Evoga::modulesMutationRate, Evoga::mutateOnlyRelatives, Evoga::mutation, Evoga::mutationdecay, Evoga::noffspring, Evoga::nogenerations, Evoga::nreplications, Evoga::numModules, Evoga::numThreads, Evoga::overwriteRate, Evoga::popSize, Evoga::randomizePop(), Evoga::rankBasedProb, Evoga::recoveredInterruptedEvolution(), Evoga::resetGenerationCounter(), Evoga::rouletteWheel(), Evoga::saveallg(), Evoga::saveallgComposed(), Evoga::saveBestTeam(), Evoga::savedConfigurationParameters, Evoga::savedExperimentPrefix, Evoga::saveFStat(), Evoga::savePopulationEachNGenerations, Evoga::saveRetStat, Evoga::saveRStat(), Evoga::selectionType, EvoRobotExperiment::setEvoga(), Evoga::setSeed(), Evoga::startingReplication(), Evoga::statfit, Evoga::targetRetentionRate, and Logger::warning().
Referenced by Evoga::evolveAllReplicas().
void evolveSteadyState | ( | ) |
Main function of the Genetic Algorithm (Steady State Version) Each individual of the population is allowed to produce an offspring that is used to replace the current worst individual or discarded depending on whether its fitness is better or not of the worst individuals During each generation individuals are re-evaluated to avoid that individuals that happened to be luck in their first evaluation continue to be overestimated with respect to their real capacities The mutation rate is initially set to 50% and then progressively reduced of 1% each generation until it reach the value specified in the mutation rate parameter This initially hight mutation rate is used to explore many random solutions during the initial generations to avoid premature convergence The size of the population is set equal to nreproducing*noffspring however, the number of offsrping is always 1 (it is independent from the noffpring parameter)
Definition at line 2382 of file evoga.cpp.
References Evoga::averageIndividualFitnessOverGenerations, Evoga::cgen, Evoga::commitStep(), Evoga::computeFStat2(), Evoga::copyGenes(), Evoga::currentSeed, EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::endGeneration(), EvoRobotExperiment::endGeneration(), Evoga::exp, Evoga::fbest, Evoga::fmin, Evoga::genome, Evoga::getCurrentSeed(), EvoRobotExperiment::getFitness(), Evoga::getGenes(), ConfigurationParameters::getObjectFromGroup(), Evoga::getPheParametersAndMutationsFromEvonet(), Evoga::getStartingSeed(), ConcurrentResourcesUser::hasResource(), Logger::info(), EvoRobotExperiment::initGeneration(), Evoga::initial_mutation, Evoga::isStopped(), Evoga::limitationFactor, Evoga::limitRetention, Evoga::loadallg(), Evoga::mutation, Evoga::mutationdecay, EvoRobotExperiment::newGASeed(), Evoga::nogenerations, Evoga::nreplications, Evoga::ntfitness, Evoga::numThreads, Evoga::popSize, Evoga::randomizePop(), Evoga::recoveredInterruptedEvolution(), Evoga::resetGenerationCounter(), Evoga::saveallg(), Evoga::saveBestInd(), Evoga::savedConfigurationParameters, Evoga::savedExperimentPrefix, Evoga::saveFStat(), Evoga::savePopulationEachNGenerations, Evoga::saveRetStat, Evoga::saveRStat(), EvoRobotExperiment::setEvoga(), EvoRobotExperiment::setNetParameters(), Evoga::setSeed(), Evoga::startingReplication(), Evoga::targetRetentionRate, Evoga::tfitness, and Logger::warning().
Referenced by Evoga::evolveAllReplicas().
|
signal |
emitted when the evolution has been finished or stopped
|
virtual |
Returns the name of the file containing the given generation for the given seed.
- Parameters
-
seed the seed from which the filename should be returned generation the generation of interest
- Returns
- the name of the file with genomes for the given seed and generation
|
virtual |
int * getBestGenes | ( | int | ind | ) |
Return a pointer to the genome of one individual stored in the bestgenome matrix.
- Parameters
-
ind The id of the individual
Definition at line 1016 of file evoga.cpp.
References Evoga::bestgenome.
|
virtual |
Returns the current generation.
- Returns
- the current generation
Definition at line 3086 of file evoga.cpp.
References Evoga::cgen.
Referenced by EvoRobotExperiment::doAllTrialsForIndividual().
|
virtual |
Returns the current mutation rate.
- Note
- this can differ from generation to generation in the case of steadyState evolution
- Returns
- the current mutation rate
Definition at line 3110 of file evoga.cpp.
References Evoga::mutation.
|
virtual |
Returns the current seed.
- Returns
- the current seed
Definition at line 3096 of file evoga.cpp.
References Evoga::currentSeed.
Referenced by EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::evolveGenerational(), Evoga::evolveSteadyState(), EvoRobotExperiment::setEvoga(), and Evoga::xnes().
|
virtual |
Returns a pointer to the EvoRobotExperiment object.
It is not guaranteed that the returned object is effectively used for evolution, in some cases it could simply be a prototype used to generate objects (via cloning) that are used to evaluate agents. Use getExperimentPool() if you want to be sure to get objects used for evaluation
- Returns
- a pointer to the EvoRobotExperiment object
Definition at line 3074 of file evoga.cpp.
References Evoga::exp.
Referenced by TestRandom::buildRandomDNA(), EvoRobotComponent::evolve(), EvoRobotViewer::EvoRobotViewer(), EvolveOperation::run(), TestRandom::runTest(), TestIndividual::runTest(), and TestCurrent::runTest().
|
virtual |
Returns a list of pointers to the EvoRobotExperiment objects used by the ga to evaluate agents.
Objects returned by this function are those effectively used to evaluate individuals (see also getEvoRobotExperiment()). Note that the list could be empty.
Definition at line 3079 of file evoga.cpp.
References Evoga::exp.
int * getGenes | ( | int | ind | ) |
Return a pointer to the genome of one individual of the current population.
- Parameters
-
ind The id of the individual
Definition at line 1008 of file evoga.cpp.
References Evoga::genome.
Referenced by Evoga::evolveGenerational(), Evoga::evolveSteadyState(), Evoga::getGenesForIndividual(), and EvaluatorThreadForEvoga::setGenotype().
|
virtual |
Returns the array of genes for the given individual.
- Parameters
-
ind the individual for whom genes are requested
- Returns
- the array of genes for the given individual
Definition at line 3135 of file evoga.cpp.
References Evoga::getGenes().
Referenced by Evoga::evolveSpecializerSteadyState(), and Evoga::evolveSpecializerSteadyState2().
void getGenome | ( | int | frombestgenome, |
int | togenome, | ||
int | mut | ||
) |
Copy a genome stored in the bestgenome matrix into the genome of the current population Apply mutation during the copy.
- Parameters
-
frombestgenome The id of the genome in the best genome matrix togenome The id of the individual that should receive the genome mut A 0/1 flag that specify whether mutation should be applied or not
Definition at line 440 of file evoga.cpp.
References Evoga::bestgenome, Evonet::DEFAULT_VALUE, Evoga::genome, Evoga::glen, Evoga::mutate(), Evoga::mutation, and Evoga::mutations.
Referenced by Evoga::mreproduce(), and Evoga::reproduce().
void getLastFStat | ( | double & | min, |
double & | max, | ||
double & | average | ||
) |
return the last value of min, max and average fitness
- Parameters
-
min in this parameter will be returned the minimum fitness max in this parameter will be returned the maximum fitness average in this parameter will be returned the average fitness
Definition at line 853 of file evoga.cpp.
References Evoga::fmin.
double getNoise | ( | double | minn, |
double | maxn | ||
) |
Unused function (to be removed)
Definition at line 388 of file evoga.cpp.
References Evoga::drand().
|
virtual |
Returns the number of generations to do.
- Returns
- the number of generations
Definition at line 3106 of file evoga.cpp.
References Evoga::nogenerations.
|
virtual |
Returns the number of replications.
- Returns
- the number of replications
Definition at line 3101 of file evoga.cpp.
References Evoga::nreplications.
Referenced by EvoRobotExperiment::doAllTrialsForIndividual().
void getPheParametersAndMutationsFromEvonet | ( | ) |
transfer .phe info inside GA
- Warning
- this function assume that the EvoRotobEvaluator has a resource named "evonet" returning a pointer to an Evonet object; it this constraint is not satisfied an exception will be raised
Definition at line 1026 of file evoga.cpp.
References Evonet::copyPheParameters(), Evonet::freeParameters(), Evonet::getMutations(), Evonet::pheFileLoaded(), Evoga::setInitialPopulation(), and Evoga::setMutations().
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
virtual |
Returns the starting seed.
- Returns
- the starting seed
Definition at line 3091 of file evoga.cpp.
References Evoga::seed.
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
bool isEnabledStepByStep | ( | ) |
return true is the step-by-step modality is enabled, false otherwise
Definition at line 3066 of file evoga.cpp.
References Evoga::isStepByStep.
bool isStopped | ( | ) |
return true if the evolution process has been stopped with stop()
This method handle also the stopping process of the evolution. In fact, it return true if the evolution process has been stopped with stop() This function return true only between the calls to stop() and to resetStop() methods:
stop(); commitStep(); // <– it will return true resetStop(); commitStep(); // <– it will return false
- Note
- resetStop() is not called automatically by the Evoga after the exit for a stop, but it's called by the EvoRobotComponent after the execution of action 'stop'; If you want to use the Evoga in your custom Component remember to call resetStop()
Definition at line 3050 of file evoga.cpp.
References Evoga::stopEvolution.
Referenced by EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSteadyState(), EvolveOperation::run(), TestOperation::run(), and Evoga::xnes().
int loadallg | ( | int | gen, |
const char * | filew | ||
) |
Load the genome of the population from a file.
- Parameters
-
gen The generation number used to create the GxS?.gen file name. If gen=0 the file name should be passed explicitly ind The filename from which the genome should be load. When gen>0 this parameter is ignored
Definition at line 872 of file evoga.cpp.
References Logger::error(), Evoga::genome, Logger::info(), Evoga::loadedIndividuals, Evoga::loadgenotype(), and Evoga::seed.
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::loadGenotypes(), and Evoga::xnes().
void loadallTeams | ( | int | gen, |
const char * | filew, | ||
QVector< QVector< int > > & | teams | ||
) |
Load the teams of the population from a file.
- Parameters
-
gen The generation number used to create the GxS?.composed.gen file name. If gen=0 the file name should be passed explicitly ind The filename from which the teams should be load. When gen>0 this parameter is ignored
Definition at line 907 of file evoga.cpp.
References Logger::error(), Logger::info(), Evoga::numModules, and Evoga::seed.
Referenced by Evoga::evolveSpecializerSteadyState2().
void loadgenotype | ( | FILE * | fp, |
int | ind | ||
) |
Load the genome of one individual in a text file.
- Parameters
-
*fp The pointer to .gen file ind The id of the individual
Definition at line 859 of file evoga.cpp.
References Evoga::genome, and Evoga::glen.
Referenced by Evoga::loadallg().
|
virtual |
Loads genotypes from the given file.
- Parameters
-
filename the name of the file to load
- Returns
- the number of individuals that have been loaded, 0 in case of errors
Definition at line 3118 of file evoga.cpp.
References Evoga::evolutionType, and Evoga::loadallg().
Referenced by TestIndividual::runTest(), and TestIndividual::setPopulationToTest().
int loadStatistics | ( | char * | filename | ) |
Load the fitness statistics from a .fit file Return the number of loaded individuals (0 if the file does not exists)
- Parameters
-
*filename The filename
Definition at line 954 of file evoga.cpp.
References farsa::max(), farsa::min(), and Evoga::statfit.
int mrand | ( | int | i | ) |
return a random integer generated with an uniform distribution in the range [0, i-1]
- Parameters
-
i The maximum value of the integer to be generated
Definition at line 308 of file evoga.cpp.
Referenced by Evoga::randomizePop(), and Evoga::setInitialPopulation().
void mreproduce | ( | ) |
Identify the best individuals and copy their genotypes into the bestgenome Matrix It also save the best genotypes in the Bx.gen files Assume that the best individuals have the lowest fitness (minimization) This function is not used and it is to be removed.
Definition at line 617 of file evoga.cpp.
References Evoga::cgen, Evoga::computeFStat(), Evoga::currentSeed, Evoga::elitism, Logger::error(), Evoga::getGenome(), Evoga::noffspring, Evoga::nogenerations, Evoga::nreproducing, Evoga::popSize, Evoga::putGenome(), Evoga::saveagenotype(), Evoga::savebest, Evoga::saveFStat(), and Evoga::tfitness.
int mutate | ( | int | w, |
double | mut | ||
) |
Mutate one gene Since genes are store as integer, this function first trasform them into 8 bits and then mutate (i.e.
flip) each bit with a certain probability
- Parameters
-
w The integer storing the gene value mut The mutation probability (i.e. the probability that each bit of the gene is flipped)
Definition at line 399 of file evoga.cpp.
References Evoga::drand().
Referenced by Evoga::copyGenes(), and Evoga::getGenome().
|
virtual |
Returns number of loaded genotypes.
- Returns
- the number of individuals that have been loaded
Definition at line 3130 of file evoga.cpp.
References Evoga::loadedIndividuals.
|
virtual |
This function is called after all linked objects have been configured.
Reimplemented from ParameterSettable.
Definition at line 2938 of file evoga.cpp.
References Evoga::bestgenome, Evoga::cgen, Evonet::DEFAULT_VALUE, Evoga::evolutionType, Evoga::exp, Evoga::genome, EvoRobotExperiment::getGenomeLength(), Evoga::glen, Logger::info(), Evoga::MAXINDIVIDUALS, Evoga::mutations, Evoga::noffspring, Evoga::nogenerations, Evoga::nreproducing, Evoga::ntfitness, Evoga::numModules, Evoga::popSize, ConcurrentResourcesUser::shareResourcesWith(), Evoga::statfit, Evoga::terror, Evoga::tfitness, ConcurrentResourcesUser::usableResources(), Evoga::xnesCombination, and Evoga::xnesCombinationType.
void printBest | ( | ) |
Print the fitness of the best individuals of the current population.
Definition at line 695 of file evoga.cpp.
References Evoga::bestgenome, Evoga::glen, and Logger::info().
void printPop | ( | ) |
Print the fitness of the individuals of the current population.
Definition at line 684 of file evoga.cpp.
References Evoga::genome, Evoga::glen, Logger::info(), Evoga::popSize, and Evoga::tfitness.
void putGenome | ( | int | fromgenome, |
int | tobestgenome | ||
) |
Copy the genome of one individual to the bestgenome matrix in a specific position.
- Parameters
-
fromgenome The id of the individual genome to be copies tobestgenome The position in which the genome should be copied
Definition at line 429 of file evoga.cpp.
References Evoga::bestgenome, Logger::error(), Evoga::genome, Evoga::glen, and Evoga::nreproducing.
Referenced by Evoga::mreproduce(), and Evoga::reproduce().
void randomizePop | ( | ) |
Randomize the genome of the population.
Definition at line 977 of file evoga.cpp.
References Evoga::genome, Evoga::glen, and Evoga::mrand().
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
signal |
emitted when an interrupted evolution has been recovered and it's going to start from there
- Parameters
-
statFile is the filename from which the evolution has been recovered
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
void reproduce | ( | ) |
Identify the best individuals and copy their genotypes into the bestgenome Matrix It also save the best genotypes in the Bx.gen files.
Definition at line 471 of file evoga.cpp.
References Evoga::cgen, Evoga::computeFStat(), Evoga::currentSeed, Evoga::elitism, Logger::error(), Evoga::getGenome(), Evoga::noffspring, Evoga::nogenerations, Evoga::nreproducing, Evoga::popSize, Evoga::putGenome(), Evoga::saveagenotype(), Evoga::savebest, Evoga::saveFStat(), and Evoga::tfitness.
Referenced by Evoga::evolveGenerational().
void resetGenerationCounter | ( | ) |
Reset the generation counter to 0.
Definition at line 1021 of file evoga.cpp.
References Evoga::cgen.
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
void resetStop | ( | ) |
reset the stopped status to false
Definition at line 3054 of file evoga.cpp.
References Evoga::stopEvolution.
Referenced by EvoRobotComponent::evolve(), EvolveOperation::run(), TestOperation::run(), and EvoRobotComponent::runTest().
|
virtual |
int rouletteWheel | ( | QVector< double > | candidates | ) |
perform a roulette wheel and return the winner's index
- Parameters
-
candidates A vector of Fitness and Id's
Definition at line 3829 of file evoga.cpp.
Referenced by Evoga::evolveSpecializerSteadyState2().
|
virtual |
Save the actual status of parameters into the ConfigurationParameters object passed.
This is not implemented, a call to this function will cause an abort
- Parameters
-
params the configuration parameters object on which save actual parameters prefix the prefix to use to access the object configuration parameters.
Implements ParameterSettable.
Definition at line 2880 of file evoga.cpp.
References Logger::error().
Referenced by EvoRobotComponent::save().
void saveagenotype | ( | FILE * | fp, |
int | ind | ||
) |
Save the genome of one individual in a text file.
- Parameters
-
*fp The pointer to .gen file ind The id of the individual
Definition at line 763 of file evoga.cpp.
References Evoga::genome, and Evoga::glen.
Referenced by Evoga::mreproduce(), Evoga::reproduce(), Evoga::saveallg(), Evoga::saveBestInd(), and Evoga::saveBestTeam().
void saveallg | ( | ) |
Save the genome of the current population in a G?S?.gen file.
Definition at line 773 of file evoga.cpp.
References Evoga::cgen, Evoga::currentSeed, Logger::error(), Evoga::popSize, and Evoga::saveagenotype().
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
void saveallgComposed | ( | QVector< QVector< int > > | composedGen | ) |
Save the composed genome of the current population in a G?S?.composed.gen file.
Definition at line 792 of file evoga.cpp.
References Evoga::cgen, Evoga::currentSeed, Logger::error(), and Evoga::numModules.
Referenced by Evoga::evolveSpecializerSteadyState2().
void saveBestInd | ( | ) |
Append the genome of the best individual of the current population to the BdSd.gen file.
Definition at line 537 of file evoga.cpp.
References Evoga::cgen, Evoga::currentSeed, Logger::error(), Evoga::ntfitness, Evoga::popSize, Evoga::saveagenotype(), and Evoga::tfitness.
Referenced by Evoga::evolveSteadyState().
void saveBestTeam | ( | QVector< QVector< int > > | teams, |
QVector< double > | fitness | ||
) |
Append the best team of the current population to the BdSd.composed.gen file.
- Parameters
-
teams Vector with the selected teams fitness Vector with the fitness of each team
Definition at line 577 of file evoga.cpp.
References Evoga::cgen, Evoga::currentSeed, Logger::error(), farsa::max(), Evoga::numModules, and Evoga::saveagenotype().
Referenced by Evoga::evolveSpecializerSteadyState2().
void saveFStat | ( | ) |
Save the average, minimal and maximal fitness by appending a line to the statSd.fit file.
Definition at line 815 of file evoga.cpp.
References Evoga::cgen, Evoga::currentSeed, Logger::error(), and Evoga::fmin.
Referenced by Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::mreproduce(), and Evoga::reproduce().
void saveRStat | ( | QVector< int > | subsVec | ) |
Save the information regarding the offspring retention by appending a line to the retention statistics file (function retentions.
- Parameters
-
subsVec A vector that has a value of -1 if the nth offspring (correspondent with the index of the vector) was not used, or the index of the parent that it tooks the place
Definition at line 832 of file evoga.cpp.
References Evoga::cgen, Evoga::currentSeed, and Logger::error().
Referenced by Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
virtual |
Set the current mutation rate to use.
- Parameters
-
mutation_rate the new mutation rate to use
Definition at line 3114 of file evoga.cpp.
References Evoga::mutation.
void setInitialPopulation | ( | int * | ge | ) |
Overwrite the genome of all individuals with a manually specify vector of parameters (loaded from a .phe file) This enable to manually fix some of the parameters The Evonet::DEFAULT_VALUE value is used to indicate the parameters are not fixed and that are replaced with randomly generated value.
- Parameters
-
int* Pointer to the vector of parameter to be used to overwrite genes
Definition at line 986 of file evoga.cpp.
References Evonet::DEFAULT_VALUE, Evoga::genome, Evoga::glen, and Evoga::mrand().
Referenced by Evoga::getPheParametersAndMutationsFromEvonet().
void setMutations | ( | float * | mut | ) |
Set the mutation rate of each parameter.
- Parameters
-
float* Pointer to the vector of mutation rate to be used
Definition at line 1001 of file evoga.cpp.
References Evoga::glen, and Evoga::mutations.
Referenced by Evoga::getPheParametersAndMutationsFromEvonet().
void setSeed | ( | int | s | ) |
Initialize the random number generator.
- Parameters
-
s The seed use dto initialize the random number generator
Definition at line 375 of file evoga.cpp.
References Evoga::currentSeed, farsa::globalRNG, and RandomGenerator::setSeed().
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), TestRandom::runTest(), TestIndividual::runTest(), TestIndividual::setPopulationToTest(), and Evoga::xnes().
|
signal |
emitted when a replication is going to start
- Parameters
-
replication is the number of the replication that it's going to start
Referenced by Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
virtual |
void stop | ( | ) |
stop the running evolution process as soon as possible
- Note
- this method return immediately, but the evolution process may take some time before to quit depending on how many processing time last a step of the evolution process
Definition at line 3035 of file evoga.cpp.
References Evoga::stopEvolution, and Evoga::waitForNextStep.
Referenced by EvolveOperation::stop(), and TestOperation::stop().
void updateGenomeFromEvonet | ( | int | ind | ) |
update the genome of the individual to match the current weights of Evonet
- Parameters
-
ind the id of the individual to update
Definition at line 1050 of file evoga.cpp.
References Evoga::genome, Evonet::getFreeParameter(), Evonet::getWrange(), and Evoga::glen.
void xnes | ( | ) |
Main function of the xNES Algorithm (exponential Natural Evolution Strategies) The size of the population is set to 1 (i.e.
there is only an individual). The individual is allowed to generate a number of offsprings (noffspring parameter) equal to L = 4 + 3 * log(glen), where <glen> is the size of genome. Every generation a new individual is obtained by calculating the direction of the expected fitness natural gradient and applying the update.
Definition at line 1299 of file evoga.cpp.
References Evoga::averageIndividualFitnessOverGenerations, Evoga::backPropOffspringFitnessIncrease, Evoga::backPropOffspringFitnessIncreasePercentage, Evoga::backPropOffspringUtilityRank, Evoga::cgen, Evoga::commitStep(), Evoga::currentSeed, Evoga::debugPrintInfoToFile, Evoga::dissociateCovMatrix, Evoga::dissociateInd, EvoRobotExperiment::doAllTrialsForIndividual(), Evoga::endGeneration(), EvoRobotExperiment::endGeneration(), Logger::error(), Evoga::exp, Evoga::fbest, Evoga::fmin, Evoga::gaussianMean, Evoga::gaussianStdDev, Evoga::getCurrentSeed(), RandomGenerator::getDouble(), EvoRobotExperiment::getError(), EvoRobotExperiment::getFitness(), Evonet::getFreeParameter(), RandomGenerator::getGaussian(), EvoRobotExperiment::getNeuralNetwork(), ConfigurationParameters::getObjectFromGroup(), Evoga::getPheParametersAndMutationsFromEvonet(), Evoga::getStartingSeed(), Evoga::glen, farsa::globalRNG, ConcurrentResourcesUser::hasResource(), Logger::info(), EvoRobotExperiment::initGeneration(), Evoga::isStopped(), Evoga::loadallg(), Evoga::minimization, Evoga::mutationLearningRate, Evoga::mutationRange, EvoRobotExperiment::newGASeed(), Evoga::nogenerations, Evoga::nreplications, Evoga::ntfitness, Evoga::numStartInd, Evoga::numThreads, Evoga::offspringMutRange, Evoga::offspringStdDev, Evoga::pheGen, Evoga::recoveredInterruptedEvolution(), Evoga::resetGenerationCounter(), Evoga::savedConfigurationParameters, Evoga::savedExperimentPrefix, Evoga::savePopulationEachNGenerations, EvoRobotExperiment::setEvoga(), EvoRobotExperiment::setNetParameters(), Evoga::setSeed(), Evoga::specialUtilityRanking, Evoga::standardNes, Evoga::startingReplication(), Evoga::terror, Evoga::tfitness, Evoga::useGaussian, Evoga::variableStdDev, Logger::warning(), Evoga::xnesCombination, and Evoga::xnesCombinationType.
Referenced by Evoga::evolveAllReplicas().
Member Data Documentation
|
protected |
Whether to average an individual fitness with its previous one or not.
The steady-state genetic algorithm original version keeps track of an individual fitness to try to reduce the effect of lucky trials. To do this the fitness obtained in generation n, f(n) is averaged with the stored fitness s(n) = 0.5 * (f(n-1) + s(n-1)). The initial condition is the fitness at the generation when the individual was first evaluated. This however causes some problems with incremental fitnesses, because if at generation i we add a new fitness component, all individuals that had a stored fitness have their fitness averaged with the old one, while new children don't
Definition at line 782 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
protected |
Flag whether to increase the fitness of the backpropagation offspring.
Definition at line 809 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
Percentage of increase of the fitness of the backpropagation offspring.
Definition at line 811 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
The utility rank of the backpropagation offspring in the second type of combination with xNES.
Definition at line 807 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
The matrix that contains a copy of the genome of the best individuals.
Definition at line 708 of file evoga.h.
Referenced by Evoga::configure(), Evoga::getBestGenes(), Evoga::getGenome(), Evoga::postConfigureInitialization(), Evoga::printBest(), and Evoga::putGenome().
|
protected |
current generation
Definition at line 745 of file evoga.h.
Referenced by Evoga::computeFStat(), Evoga::computeFStat2(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getCurrentGeneration(), Evoga::mreproduce(), Evoga::postConfigureInitialization(), Evoga::reproduce(), Evoga::resetGenerationCounter(), Evoga::saveallg(), Evoga::saveallgComposed(), Evoga::saveBestInd(), Evoga::saveBestTeam(), Evoga::saveFStat(), Evoga::saveRStat(), and Evoga::xnes().
|
protected |
The probability that a crossover will occur in an offspring team – specializerSteadyState.
Definition at line 834 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::evolveSpecializerSteadyState2().
|
protected |
The seed of the current generation (used for example to generate the name of the G?S?.gen files)
Definition at line 733 of file evoga.h.
Referenced by Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getCurrentSeed(), Evoga::mreproduce(), Evoga::reproduce(), Evoga::saveallg(), Evoga::saveallgComposed(), Evoga::saveBestInd(), Evoga::saveBestTeam(), Evoga::saveFStat(), Evoga::saveRStat(), Evoga::setSeed(), and Evoga::xnes().
|
protected |
Flags whether to print debug information about the xNES algorithm to file.
Definition at line 819 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
Flags whether to dissociate back-propagation effect on the xNES covariance matrix update.
Definition at line 823 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
Flags whether to dissociate back-propagation effect on the xNES individual update.
Definition at line 821 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
if the Evoga will use elitism or not
Definition at line 604 of file evoga.h.
Referenced by Evoga::configure(), Evoga::mreproduce(), and Evoga::reproduce().
|
protected |
specify the type of the evolution process to execute This string can only assume two values at the moment: 'steadyState' or 'generational' or 'xnes'
Definition at line 725 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveAllReplicas(), Evoga::loadGenotypes(), and Evoga::postConfigureInitialization().
|
protected |
Definition at line 598 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getEvoRobotExperiment(), Evoga::getEvoRobotExperimentPool(), Evoga::postConfigureInitialization(), Evoga::xnes(), and Evoga::~Evoga().
|
protected |
fitness best
Definition at line 751 of file evoga.h.
Referenced by Evoga::computeFStat(), Evoga::computeFStat2(), Evoga::evolveGenerational(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
protected |
fitness best generation (maximum)
Definition at line 753 of file evoga.h.
Referenced by Evoga::computeFStat(), and Evoga::computeFStat2().
|
protected |
fitness statistics (minimum, maximum, and average fitness)
Definition at line 749 of file evoga.h.
Referenced by Evoga::computeFStat(), Evoga::computeFStat2(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getLastFStat(), Evoga::saveFStat(), and Evoga::xnes().
|
protected |
Mean of the gaussian distribution.
Definition at line 791 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
Standard deviation of the gaussian distribution.
Definition at line 793 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
The matrix that contain the genome of the corrent population.
Definition at line 706 of file evoga.h.
Referenced by Evoga::configure(), Evoga::copyGenes(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getGenes(), Evoga::getGenome(), Evoga::loadallg(), Evoga::loadgenotype(), Evoga::postConfigureInitialization(), Evoga::printPop(), Evoga::putGenome(), Evoga::randomizePop(), Evoga::saveagenotype(), Evoga::setInitialPopulation(), and Evoga::updateGenomeFromEvonet().
|
protected |
genome lenght (number of free parameters)
Definition at line 602 of file evoga.h.
Referenced by Evoga::copyGenes(), Evoga::getGenome(), Evoga::loadgenotype(), Evoga::postConfigureInitialization(), Evoga::printBest(), Evoga::printPop(), Evoga::putGenome(), Evoga::randomizePop(), Evoga::saveagenotype(), Evoga::setInitialPopulation(), Evoga::setMutations(), Evoga::updateGenomeFromEvonet(), and Evoga::xnes().
|
protected |
The initial mutation rate setted when steadyState algorithm is used.
Definition at line 741 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
protected |
if step-by-step modality is enabled or not
Definition at line 759 of file evoga.h.
Referenced by Evoga::commitStep(), Evoga::enableStepByStep(), and Evoga::isEnabledStepByStep().
|
protected |
The limitation factor that will multiply the offsprings' fitness.
Definition at line 854 of file evoga.h.
Referenced by Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
protected |
Wheather the retention should be limited or not.
Definition at line 831 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
protected |
The number of individual genome loaded from a .gen file into the genome matrix (-1 when none has been loaded)
Definition at line 712 of file evoga.h.
Referenced by Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::loadallg(), and Evoga::numLoadedGenotypes().
|
static |
constant defining the maximimum number of individuals that Evoga can manage
Definition at line 73 of file evoga.h.
Referenced by Evoga::postConfigureInitialization().
|
protected |
Flags whether the fitness function is set to solve a minimization problem.
Definition at line 817 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
The probability that a module will be mutated on a team – specializerSteadyState.
Definition at line 837 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::evolveSpecializerSteadyState2().
|
protected |
Wheater a module should be replace by its own offspring or by any offspring – specializerSteadyState.
Definition at line 840 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::evolveSpecializerSteadyState2().
|
protected |
The mutation rate can vary from 0 to 1 continuosly to express probability in the range [0, 100%].
Definition at line 735 of file evoga.h.
Referenced by Evoga::configure(), Evoga::copyGenes(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getCurrentMutationRate(), Evoga::getGenome(), and Evoga::setCurrentMutationRate().
|
protected |
The amount of reduction of the mutation generations during the first generations until it reaches the specified mutation rate.
Definition at line 739 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
protected |
The mutation learning rate used to generate offspring from backprop array.
Definition at line 801 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
The mutation range used in XNES algorithm to initialize the individual.
Definition at line 785 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
vector of parameter-specific mutations
Definition at line 743 of file evoga.h.
Referenced by Evoga::copyGenes(), Evoga::getGenome(), Evoga::postConfigureInitialization(), Evoga::setMutations(), and Evoga::~Evoga().
|
protected |
the mutex used for controlling the step-by-step modality
Definition at line 761 of file evoga.h.
Referenced by Evoga::commitStep().
|
protected |
number of sons
Definition at line 608 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState2(), Evoga::mreproduce(), Evoga::postConfigureInitialization(), and Evoga::reproduce().
|
protected |
The number of generations specified with the ngenerations parameter.
Definition at line 727 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getNumOfGenerations(), Evoga::mreproduce(), Evoga::postConfigureInitialization(), Evoga::reproduce(), Evoga::xnes(), and Evoga::~Evoga().
|
protected |
The number of replications specified with the corresponding parameter.
Definition at line 729 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::getNumReplications(), and Evoga::xnes().
|
protected |
number of individuals allowed to reproduce
Definition at line 606 of file evoga.h.
Referenced by Evoga::configure(), Evoga::mreproduce(), Evoga::postConfigureInitialization(), Evoga::putGenome(), and Evoga::reproduce().
|
protected |
A pointer to a vector used to store the total number of trials in which an unchanged individuals have been evaluated.
Definition at line 716 of file evoga.h.
Referenced by Evoga::computeFStat2(), Evoga::evolveSteadyState(), Evoga::postConfigureInitialization(), Evoga::saveBestInd(), Evoga::xnes(), and Evoga::~Evoga().
int numModules |
The number of modules that a genotype should use.
Definition at line 582 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveAllReplicas(), Evoga::evolveSpecializerSteadyState2(), Evoga::loadallTeams(), Evoga::postConfigureInitialization(), Evoga::saveallgComposed(), and Evoga::saveBestTeam().
|
protected |
Number of possible individuals from which finding out the starting individual of XNES.
Definition at line 799 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
The number of concurrent threads to use.
Definition at line 765 of file evoga.h.
Referenced by Evoga::configure(), Evoga::doNotUseMultipleThreads(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
protected |
The mutation range used in XNES algorithm to generate the offspring.
Definition at line 787 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
Standard deviation to generate offspring.
Definition at line 795 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
The probability that a module will be overwritten by another one – specializerSteadyState.
Definition at line 849 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::evolveSpecializerSteadyState2().
|
protected |
How often (in terms of number of generations) the best phenotype must be saved.
Definition at line 813 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
population size
Definition at line 600 of file evoga.h.
Referenced by Evoga::computeFStat(), Evoga::computeFStat2(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), Evoga::mreproduce(), Evoga::postConfigureInitialization(), Evoga::printPop(), Evoga::reproduce(), Evoga::saveallg(), and Evoga::saveBestInd().
|
protected |
The probability that the individuals with the highest fitness will be selected.
Definition at line 843 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::evolveSpecializerSteadyState2().
|
protected |
save best savebest parents by default equals 1
Definition at line 747 of file evoga.h.
Referenced by Evoga::configure(), Evoga::mreproduce(), and Evoga::reproduce().
|
protected |
A copy of the ConfigurationParameters object that was passed to our configure method.
We need this to create other instances of the experiment
Definition at line 862 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
protected |
A copy of the prefix for experiment parameters.
We need this to create other instances of the experiment
Definition at line 869 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
protected |
How often (i.e. how many generations) we want to same the population genome in a .gen file.
Definition at line 767 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveGenerational(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::evolveSteadyState(), and Evoga::xnes().
|
protected |
Whether to save retentions statistics or not.
Definition at line 828 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
protected |
The number used to initialized the seed of the first replication (successive replication use incremented numbers)
Definition at line 731 of file evoga.h.
Referenced by Evoga::configure(), Evoga::getStartingSeed(), Evoga::loadallg(), and Evoga::loadallTeams().
|
protected |
The type of selection that will be used to define which individuals will survive.
Definition at line 846 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::evolveSpecializerSteadyState2().
|
protected |
Flags whether to use a special version to compute utility ranking.
Definition at line 825 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
Flags whether the standard xNES algorithm must be used (i.e. the parameters are set according to the paper)
Definition at line 815 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
A matrix that store the statistics (average, min, and max fitness) for all generations.
Definition at line 718 of file evoga.h.
Referenced by Evoga::computeFStat(), Evoga::computeFStat2(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), Evoga::loadStatistics(), Evoga::postConfigureInitialization(), and Evoga::~Evoga().
|
protected |
Flag used to request to end the evolutionary process.
Definition at line 757 of file evoga.h.
Referenced by Evoga::commitStep(), Evoga::evolveAllReplicas(), Evoga::isStopped(), Evoga::resetStop(), and Evoga::stop().
|
protected |
The target retention rate.
Definition at line 852 of file evoga.h.
Referenced by Evoga::configure(), Evoga::evolveSpecializerSteadyState(), Evoga::evolveSpecializerSteadyState2(), and Evoga::evolveSteadyState().
|
protected |
A pointer to a vector used to store the sum of the errors obtained during different evaluations of an unmodified individual.
Definition at line 720 of file evoga.h.
Referenced by Evoga::postConfigureInitialization(), Evoga::xnes(), and Evoga::~Evoga().
|
protected |
A pointer to a vector used to store the sum of the fitness obtained during different evaluations of an unmodified individual.
Definition at line 714 of file evoga.h.
Referenced by Evoga::computeFStat(), Evoga::computeFStat2(), Evoga::evolveGenerational(), Evoga::evolveSteadyState(), Evoga::mreproduce(), Evoga::postConfigureInitialization(), Evoga::printPop(), Evoga::reproduce(), Evoga::saveBestInd(), Evoga::xnes(), and Evoga::~Evoga().
|
protected |
Flag to decide whether using gaussian distribution to generate offspring.
Definition at line 789 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
Flags whether the standard deviation to generate offspring is constant or not.
Definition at line 797 of file evoga.h.
Referenced by Evoga::configure(), and Evoga::xnes().
|
protected |
the wait condition for waiting on step-by-step modality
Definition at line 763 of file evoga.h.
Referenced by Evoga::commitStep(), Evoga::doNextStep(), Evoga::enableStepByStep(), and Evoga::stop().
|
protected |
Flag to decide whether combining xnes with backpropagation algorithm.
Definition at line 803 of file evoga.h.
Referenced by Evoga::configure(), Evoga::postConfigureInitialization(), and Evoga::xnes().
|
protected |
The type of combination between xnes and backpropagation algorithms.
Definition at line 805 of file evoga.h.
Referenced by Evoga::configure(), Evoga::postConfigureInitialization(), and Evoga::xnes().
The documentation for this class was generated from the following files: