The linear camera sensor of the e-puck. More...
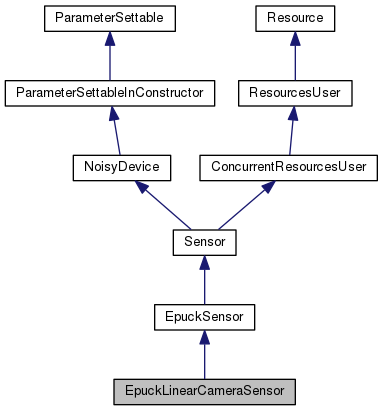
Public Member Functions | |
EpuckLinearCameraSensor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~EpuckLinearCameraSensor () |
Destructor. More... | |
const LinearCamera * | getCamera () const |
Returns the camera sensor used internally. More... | |
int | getNumReceptors () const |
Returns the number of receptors of the camera. More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Saves current parameters into the given ConfigurationParameters object. More... | |
virtual int | size () |
Returns the number of neurons required by this sensor. More... | |
virtual void | update () |
Performs the sensor update. This also modifies the activation of input neurons. More... | |
![]() | |
EpuckSensor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~EpuckSensor () |
Destructor. More... | |
![]() | |
Sensor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~Sensor () | |
Destructor. More... | |
QString | name () |
Return the name of the Sensor. More... | |
void | save (ConfigurationParameters ¶ms, QString prefix) |
Save the parameters into the ConfigurationParameters. More... | |
void | setName (QString name) |
Use this method for changing the name of the Sensor. More... | |
![]() | |
NoisyDevice (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~NoisyDevice () | |
Destructor. More... | |
![]() | |
ParameterSettableInConstructor (ConfigurationParameters &, QString) | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
virtual void | postConfigureInitialization () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
![]() | |
void | addUsableResource (QString resource) |
void | addUsableResources (QStringList resources) |
void | declareResource (QString name, T *resource, QString lockBuddy="") |
void | deleteResource (QString name) |
T * | getResource (QString name, bool *resourceExists=NULL) |
bool | hasResource (QString name) const |
void | removeAllUsableResources () |
void | removeUsableResource (QString resource) |
void | removeUsableResources (QStringList resources) |
virtual void | shareResourcesWith (ResourcesUser *buddy) |
void | usableResources (QStringList resources) |
bool | usedResourcesExist (QStringList *nonExistingResources=NULL) const |
Static Public Member Functions | |
static void | describe (QString type) |
Generates a description of this class and its parameters. More... | |
![]() | |
static void | describe (QString type) |
Describes all the parameters for this sensor. More... | |
![]() | |
static void | describe (QString type) |
Describe all the parameter for configuring the Sensor. More... | |
![]() | |
static void | describe (QString type) |
Describe all the parameter for configuring the Sensor. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
typedef Resource::ResourceChangeType | ResourceChangeType |
![]() | |
enum | ResourceChangeType |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
QString | actualResourceNameForMultirobot (QString resourceName) const |
Returns the actual resource name to use. More... | |
void | checkAllNeededResourcesExist () |
Checks whether all resources we need are existing and throws an exception if they aren't. More... | |
void | resetNeededResourcesCheck () |
Resets the check on needed resources so that the next call to checkAllNeededResourcesExist() will perform the full check and not the quick one. More... | |
![]() | |
double | applyNoise (double v, double minValue, double maxValue) const |
Adds noise to the value. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
![]() | |
T * | getResource () |
![]() | |
ResourcesUser (const ResourcesUser &other) | |
virtual void | notifyResourceChange (ResourceHandler *resource, ResourceChangeType changeType)=0 |
ResourcesUser & | operator= (const ResourcesUser &other) |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
![]() | |
QString | m_epuckResource |
The name of the resource associated with the e-puck robot. More... | |
QString | m_neuronsIteratorResource |
The name of th resource associated with the neural network iterator. More... | |
![]() | |
ResourceCollectionHolder | m_resources |
Detailed Description
The linear camera sensor of the e-puck.
This is a linear camera with configurable aperture (using the aperture parameter). The number of photoreceptors can be configured using the numReceptors parameter. Each receptor returns three values, one for each of the three colors (red, green, blue). This means that the size returned by this sensor is 3 * numReceptors. The inputs for the controller are returned in the following order: first the values of all the red receptors, then the values for all the green receptors, finally the values for all the blue receptors. This sensor only works with objects created using the Arena instance. This sensor applies noise if requested
In addition to all parameters defined by the parent class (EpuckSensor), this class also defines the following parameters:
- numReceptors: the number of receptors of the sensor. Each receptor returns three values, one for each of the three colors (red, green, blue). This means that the size returned by this sensor is 3 * numReceptors (default is 8)
- aperture: the aperture of the camera in degrees. The real e-puck has a camera with an aperture of 36°, but here you can use any value up to 360°
- drawCamera: whether to draw the camera on the robot or not (default is true)
- ignoreWalls: whether to perceive walls or not (default is false)
The resources required by this Sensor are the same as those of the parent class plus:
- arena: the instance of the Arena object where the robot lives
Definition at line 318 of file epucksensors.h.
Constructor & Destructor Documentation
EpuckLinearCameraSensor | ( | ConfigurationParameters & | params, |
QString | prefix | ||
) |
Constructor.
Creates and configures the sensor
- Parameters
-
params the ConfigurationParameters containing the parameters prefix the path prefix to the paramters for this Sensor
Definition at line 245 of file epucksensors.cpp.
|
virtual |
Destructor.
Definition at line 257 of file epucksensors.cpp.
Member Function Documentation
|
static |
Generates a description of this class and its parameters.
- Parameters
-
type the string representation of this class name
Definition at line 276 of file epucksensors.cpp.
References ParameterSettable::addTypeDescription(), EpuckSensor::describe(), and ParameterSettable::MaxInteger.
|
inline |
Returns the camera sensor used internally.
- Returns
- the camera sensor used internally
Definition at line 380 of file epucksensors.h.
|
inline |
Returns the number of receptors of the camera.
- Returns
- the number of receptors of the camera
Definition at line 370 of file epucksensors.h.
|
virtual |
Saves current parameters into the given ConfigurationParameters object.
- Parameters
-
params the ConfigurationParameters object in which parameters should be saved prefix the name and path of the group where to save parametrs
Reimplemented from EpuckSensor.
Definition at line 263 of file epucksensors.cpp.
References ConfigurationParameters::createParameter(), EpuckSensor::save(), and ConfigurationParameters::startObjectParameters().
|
virtual |
Returns the number of neurons required by this sensor.
- Returns
- the number of neurons required by this sensor
Implements Sensor.
Definition at line 313 of file epucksensors.cpp.
|
virtual |
Performs the sensor update. This also modifies the activation of input neurons.
Implements Sensor.
Definition at line 289 of file epucksensors.cpp.
References NoisyDevice::applyNoise(), Sensor::checkAllNeededResourcesExist(), LinearCamera::colorForReceptor(), Sensor::name(), NeuronsIterator::nextNeuron(), NeuronsIterator::setCurrentBlock(), NeuronsIterator::setInput(), and LinearCamera::update().
The documentation for this class was generated from the following files:
- experiments/include/epucksensors.h
- experiments/src/epucksensors.cpp