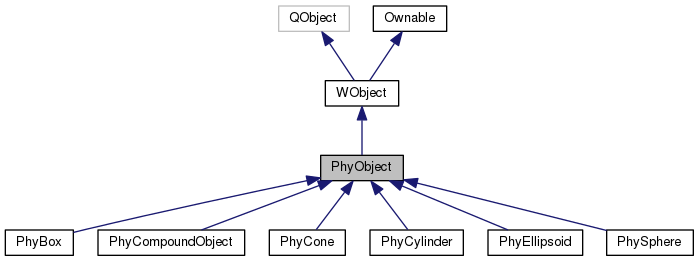
Public Member Functions | |
PhyObject (World *world, QString name="unamed", const wMatrix &tm=wMatrix::identity(), bool cp=true) | |
Create a physics object with no-collision-shape and insert it in the world passed. More... | |
virtual | ~PhyObject () |
Destroy this object. More... | |
void | addForce (const wVector &force) |
void | addImpulse (const wVector &pointDeltaVeloc, const wVector &pointPosit) |
void | addTorque (const wVector &torque) |
wVector | force () |
bool | getKinematic () const |
Returns true if the object has kinematic behaviour. More... | |
bool | getStatic () const |
Returns true if the object is static. More... | |
wVector | inertiaVec () const |
wVector | invInertiaVec () const |
Return the inverse of Inertia. More... | |
wVector | invMassInertiaVec () const |
bool | isCollidable () const |
Returns true if this object collides with other object. More... | |
real | mass () |
Return the mass. More... | |
wVector | massInertiaVec () const |
Return the Mass and momentum of Inertia. More... | |
QString | material () const |
wVector | omega () |
void | reset () |
reset the object: set the velocity to zeroset the angural velocity to zeroset any residual forces and torques to zeroremove any pending collisionMore... | |
void | setForce (const wVector &force) |
void | setKinematic (bool b, bool c=false) |
Changes between kinematic/dynamic behaviour for the object. More... | |
void | setMass (real) |
Set the mass without touching the Inertia data. More... | |
void | setMassInertiaVec (const wVector &) |
Set the Mass and momentum of Inertia: ( mass, Ixx, Iyy, Izz ) More... | |
void | setMaterial (QString material) |
void | setOmega (const wVector &omega) |
void | setStatic (bool b) |
Makes the object static or not. More... | |
void | setTorque (const wVector &torque) |
void | setVelocity (const wVector &velocity) |
wVector | torque () |
wVector | velocity () |
![]() | |
WObject (World *world, QString name="unamed", const wMatrix &tm=wMatrix::identity(), bool addToWorld=true) | |
create the object and automatically put this into the world More... | |
virtual | ~WObject () |
destroy the Object and drop it from the world More... | |
QColor | color () const |
return the color of this object More... | |
void | drawLocalAxes (bool d) |
Sets whether the object local frame of reference should be drawn or not. More... | |
bool | isInvisible () const |
return if it is invisible More... | |
const QString & | label () const |
Returns the text label to render along with the object. More... | |
const QColor & | labelColor () const |
Returns the color of the label. More... | |
const wVector & | labelPosition () const |
Returns the label position relative to this object. More... | |
bool | labelShown () const |
Returns whether the label is shown or not. More... | |
bool | localAxesDrawn () const |
Returns true if the local frame of refecence of the object should be drawn. More... | |
const wMatrix & | matrix () const |
return a reference to the transformation matrix More... | |
QString | name () const |
Return the name of this object. More... | |
virtual void | postUpdate () |
postUpdate the WObject this method is called at each step of the world just after the physic update More... | |
virtual void | preUpdate () |
preUpdate the WObject this method is called at each step of the world just before the physic update More... | |
void | setAlpha (int alpha) |
set the value of alpha channel (the transparency) More... | |
void | setColor (QColor c) |
Set the color to use on rendering. More... | |
void | setInvisible (bool b) |
set invisibility More... | |
void | setLabel (QString label) |
Sets a text label to render along with the object. More... | |
void | setLabel (QString label, wVector pos) |
Sets a text label to render along with the object and its position. More... | |
void | setLabel (QString label, wVector pos, QColor color) |
Sets a text label to render along with the object and its position and color. More... | |
void | setLabelColor (const QColor &color) |
Sets the color of the label. More... | |
void | setLabelPosition (const wVector &pos) |
Sets the label position relative to this object. More... | |
void | setMatrix (const wMatrix &newm) |
set a new matrix More... | |
void | setPosition (const wVector &newpos) |
set the position specified in global coordinate frame More... | |
void | setPosition (real x, real y, real z) |
set the position specified in global coordinate frame More... | |
void | setTexture (QString textureName) |
Set the texture to use for this WObject when rendered. More... | |
void | setUseColorTextureOfOwner (bool b) |
set if the object will be rendered with the color and texture of our owner (if we have one) More... | |
void | showLabel (bool show) |
Sets whether to show the label or not. More... | |
QString | texture () const |
Return the texture name. More... | |
bool | useColorTextureOfOwner () const |
if true, we will use color and texture of our owner (if we have one) More... | |
World * | world () |
Return the world. More... | |
const World * | world () const |
Return the world (const version) More... | |
![]() | |
Ownable () | |
Constructor. More... | |
virtual | ~Ownable () |
Destructor. More... | |
const QList< Owned > & | owned () const |
Returns the list of objects owned by this one. More... | |
Ownable * | owner () const |
Returns the owner of this object. More... | |
void | setOwner (Ownable *owner, bool destroy=true) |
Sets the owner of this object. More... | |
Protected Member Functions | |
virtual void | changedMatrix () |
syncronize this object with underlying physic object More... | |
void | createPrivateObject () |
Protected Attributes | |
PhyObjectPrivate * | priv |
Engine encapsulation. More... | |
WorldPrivate * | worldpriv |
![]() | |
QColor | colorv |
Color, it contains also alpha channel. More... | |
bool | invisible |
if TRUE it will not renderized More... | |
QColor | labelcol |
The color of the label. More... | |
bool | labeldrawn |
Whether the label should be rendered or not. More... | |
wVector | labelpos |
The position of the label in the object frame of reference. More... | |
QString | labelv |
The text label of the object. More... | |
bool | localFrameOfReferenceDrawn |
If true, the local frame of reference of the object is drawn. More... | |
QString | namev |
Name of the WObject. More... | |
QString | texturev |
Texture name. More... | |
wMatrix | tm |
Trasformation matrix. More... | |
bool | usecolortextureofowner |
if true, we will use color and texture of our owner (if we have one). More... | |
World * | worldv |
World. More... | |
Friends | |
class | PhyCompoundObject |
class | PhyJoint |
class | PhyObjectPrivate |
class | World |
class | WorldPrivate |
Additional Inherited Members | |
![]() | |
typedef QList< Owned > | OwnedList |
The type for the list of owned objects. More... | |
Detailed Description
PhyObject class.
\
- Motivation
- The PhyObject represent a physical object.
- Description
- Warnings
- Warnings
Definition at line 46 of file phyobject.h.
Constructor & Destructor Documentation
PhyObject | ( | World * | world, |
QString | name = "unamed" , |
||
const wMatrix & | tm = wMatrix::identity() , |
||
bool | cp = true |
||
) |
Create a physics object with no-collision-shape and insert it in the world passed.
- Parameters
-
world The World which object will be inserted tm rotation and position at the moment of creation cp is for internal use; ignore it
Definition at line 26 of file phyobject.cpp.
References PhyObject::changedMatrix(), and PhyObject::priv.
|
virtual |
Member Function Documentation
|
protectedvirtual |
syncronize this object with underlying physic object
Reimplemented from WObject.
Definition at line 145 of file phyobject.cpp.
References PhyObject::priv, and WObject::tm.
Referenced by PhyBox::PhyBox(), PhyCompoundObject::PhyCompoundObject(), PhyCone::PhyCone(), PhyCylinder::PhyCylinder(), PhyEllipsoid::PhyEllipsoid(), PhyObject::PhyObject(), and PhySphere::PhySphere().
|
inline |
Returns true if the object has kinematic behaviour.
- Returns
- true if the object has kinematic behaviour
Definition at line 72 of file phyobject.h.
Referenced by World::smartCheckContacts().
|
inline |
Returns true if the object is static.
- Returns
- true if the object is static
Definition at line 97 of file phyobject.h.
Referenced by World::smartCheckContacts().
wVector invInertiaVec | ( | ) | const |
Return the inverse of Inertia.
Definition at line 210 of file phyobject.cpp.
|
inline |
Returns true if this object collides with other object.
To collide with other objects, this must be non-kinematic or, if it is, setKinematic must have been called with the c parameter set to true
- Returns
- true if this object collides with other object.
Definition at line 82 of file phyobject.h.
real mass | ( | ) |
Return the mass.
Definition at line 243 of file phyobject.cpp.
Referenced by PhyCompoundObject::createPrivateObject().
wVector massInertiaVec | ( | ) | const |
Return the Mass and momentum of Inertia.
Definition at line 193 of file phyobject.cpp.
void reset | ( | ) |
reset the object:
set the velocity to zero
set the angural velocity to zero
set any residual forces and torques to zero
remove any pending collision
Definition at line 120 of file phyobject.cpp.
References PhyObject::priv.
void setKinematic | ( | bool | b, |
bool | c = false |
||
) |
Changes between kinematic/dynamic behaviour for the object.
A kinematic object doesn't interact at all with other objects; it can only be moved directly setting its transformation matrix. By default the object is dynamic
- Parameters
-
b if true the object is set to kinematic behaviour c if true the object collides with other objects even in kinematic mode, influencing them without being influenced (technically, we set its mass to 0 in Newton Game Dynamics engine)
Definition at line 56 of file phyobject.cpp.
References PhyObject::priv, and WObject::tm.
Referenced by PhyKhepera::doKinematicSimulation(), PhyEpuck::doKinematicSimulation(), and PhyMarXbot::doKinematicSimulation().
void setMass | ( | real | newmass | ) |
Set the mass without touching the Inertia data.
Definition at line 219 of file phyobject.cpp.
References PhyObject::priv.
Referenced by PhySphere::createPrivateObject(), PhyCone::createPrivateObject(), PhyCompoundObject::createPrivateObject(), PhyEllipsoid::createPrivateObject(), PhyBox::createPrivateObject(), PhyCylinder::createPrivateObject(), PhyMarXbot::enableAttachmentDevice(), PhyEpuck::PhyEpuck(), PhyKhepera::PhyKhepera(), and PhyMarXbot::PhyMarXbot().
void setMassInertiaVec | ( | const wVector & | mi | ) |
Set the Mass and momentum of Inertia: ( mass, Ixx, Iyy, Izz )
Definition at line 184 of file phyobject.cpp.
References PhyObject::priv.
void setStatic | ( | bool | b | ) |
Makes the object static or not.
A static object is unaffected by external forces
- Parameters
-
b if true the object is static
Definition at line 107 of file phyobject.cpp.
References PhyObject::priv.
Member Data Documentation
|
protected |
Engine encapsulation.
Definition at line 159 of file phyobject.h.
Referenced by PhyObject::changedMatrix(), World::checkContacts(), World::closestPoints(), World::collisionRayCast(), PhySphere::createPrivateObject(), PhyCone::createPrivateObject(), PhyCompoundObject::createPrivateObject(), PhyEllipsoid::createPrivateObject(), PhyBox::createPrivateObject(), PhyCylinder::createPrivateObject(), PhyJoint::PhyJoint(), PhyObject::PhyObject(), PhyObject::reset(), PhyObject::setKinematic(), PhyObject::setMass(), PhyObject::setMassInertiaVec(), PhyObject::setStatic(), and PhyObject::~PhyObject().
The documentation for this class was generated from the following files:
- worldsim/include/phyobject.h
- worldsim/src/phyobject.cpp