In a BiasedCluster each neuron has an input, an output and a bias value. More...
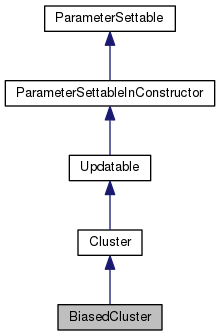
Public Member Functions | |
BiasedCluster (unsigned int numNeurons, QString name="unnamed") | |
Construct a Cluster that contains numNeurons neurons. More... | |
BiasedCluster (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~BiasedCluster () |
Destructor. More... | |
DoubleVector & | biases () |
Get the array of biases, this returns the actual array not a copy. More... | |
const DoubleVector & | biases () const |
Get the array of biases, this returns the actual array not a copy. More... | |
double | getBias (unsigned int neuron) |
Get bias of the neuron. More... | |
void | randomize (double min, double max) |
Randomize the biases of BiasedCluster. More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Save the actual status of parameters into the ConfigurationParameters object passed. More... | |
void | setAllBiases (double bias) |
Set all biases with the same value. More... | |
void | setBias (unsigned int neuron, double bias) |
Set the bias of the neuron. More... | |
void | setBiases (const DoubleVector &biases) |
Set the biases from the vector given. More... | |
void | update () |
Update the outputs of neurons. More... | |
![]() | |
Cluster (unsigned int numNeurons, QString name="unnamed") | |
Construct a Cluster. More... | |
Cluster (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~Cluster () |
Destructor. More... | |
getStateVectorFuncPtr | getDelegateFor (QString stateVector) |
Return the pointer to function for retrieving the DoubleVector representing the state requested. More... | |
double | getInput (unsigned int neuron) const |
Get the input of neuron. More... | |
double | getOutput (unsigned int neuron) const |
Get the output of neuron. More... | |
DoubleVector & | inputs () |
Get the array of inputs. More... | |
const DoubleVector & | inputs () const |
Get the array of inputs. More... | |
bool | isAccumulate () const |
return true if the Cluster will accumulates inputs More... | |
bool | needReset () |
Return true if inputs needs a reset. More... | |
unsigned int | numNeurons () const |
Return the number of neurons (the length of input and output arrays) More... | |
OutputFunction * | outFunction () const |
Get the Output function. More... | |
DoubleVector & | outputs () |
Get the array of outputs. More... | |
const DoubleVector & | outputs () const |
Get the array of outputs. More... | |
void | resetInputs () |
Reset the inputs of this cluster; the inputs will be set to zero. More... | |
void | setAccumulate (bool mode) |
Enable/Disable accumulation mode If accumulation is enabled (true) then linkers attached to this Cluster will never resetInput and accumulates data, otherwise the inputs will be resetted at each step of neural network. More... | |
void | setAllInputs (double value) |
Set all the inputs with the same value Details... More... | |
void | setInput (unsigned int neuron, double value) |
Set the input of neuron Details... More... | |
void | setInputs (const DoubleVector &inputs) |
Set the inputs from the vector given. More... | |
void | setOutFunction (OutputFunction *up) |
Set the output function for all neurons contained This method create an internal copy of the OutputFunction passed More... | |
void | setOutput (unsigned int neuron, double value) |
Force the output of the neuron at value specified. More... | |
void | setOutputs (const DoubleVector &outputs) |
Set the outputs from the vector given. More... | |
![]() | |
Updatable (QString name="unnamed") | |
Constructor. More... | |
Updatable (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~Updatable () |
Destructor. More... | |
QString | name () const |
Return its name. More... | |
void | setName (QString newname) |
Set the name of Updatable. More... | |
![]() | |
ParameterSettableInConstructor (ConfigurationParameters &, QString) | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
virtual void | postConfigureInitialization () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
Static Public Member Functions | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Additional Inherited Members | |
![]() | |
typedef DoubleVector &(* | getStateVectorFuncPtr) (Cluster *) |
Delegate type for retrieving a vector by name (pointer to function) It works in this way: More... | |
![]() | |
enum | Property |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
template<class T , DoubleVector &(T::*)() TMethod> | |
void | setDelegateFor (QString vectorName) |
Configure a delegate for a specifing state vector; who implements subclasses of Cluster has to specify a name and the method used to retrieve any state vector that needs to be public (i.e. More... | |
void | setNeedReset (bool b) |
Set the state of 'needReset' Used by subclasses into update implementation. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
Detailed Description
In a BiasedCluster each neuron has an input, an output and a bias value.
All neurons in the Cluster have the same transfer function which is given to the constructor by the outfunction property (see API doc).
Definition at line 41 of file biasedcluster.h.
Constructor & Destructor Documentation
BiasedCluster | ( | unsigned int | numNeurons, |
QString | name = "unnamed" |
||
) |
Construct a Cluster that contains numNeurons neurons.
Definition at line 29 of file biasedcluster.cpp.
BiasedCluster | ( | ConfigurationParameters & | params, |
QString | prefix | ||
) |
Constructor.
Definition at line 37 of file biasedcluster.cpp.
References ConfigurationHelper::getVector(), and Cluster::numNeurons().
|
virtual |
Destructor.
Definition at line 55 of file biasedcluster.cpp.
Member Function Documentation
|
inline |
Get the array of biases, this returns the actual array not a copy.
This allows you to change the biases using the pointer returned!
Definition at line 62 of file biasedcluster.h.
|
inline |
Get the array of biases, this returns the actual array not a copy.
This allows you to change the biases using the pointer returned!
Definition at line 68 of file biasedcluster.h.
|
static |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups.
Definition at line 98 of file biasedcluster.cpp.
References ParameterSettable::addTypeDescription(), Cluster::describe(), and ParameterSettable::IsList.
double getBias | ( | unsigned int | neuron | ) |
Get bias of the neuron.
Definition at line 76 of file biasedcluster.cpp.
|
virtual |
Randomize the biases of BiasedCluster.
Implements Cluster.
Definition at line 80 of file biasedcluster.cpp.
References RandomGenerator::getDouble(), farsa::globalRNG, and Cluster::numNeurons().
|
virtual |
Save the actual status of parameters into the ConfigurationParameters object passed.
- Parameters
-
params the configuration parameters object on which save actual parameters prefix the prefix to use to access the object configuration parameters.
Reimplemented from Cluster.
Definition at line 86 of file biasedcluster.cpp.
References ConfigurationParameters::createParameter(), Cluster::save(), and ConfigurationParameters::startObjectParameters().
void setAllBiases | ( | double | bias | ) |
Set all biases with the same value.
Definition at line 68 of file biasedcluster.cpp.
void setBias | ( | unsigned int | neuron, |
double | bias | ||
) |
Set the bias of the neuron.
Definition at line 64 of file biasedcluster.cpp.
void setBiases | ( | const DoubleVector & | biases | ) |
Set the biases from the vector given.
Definition at line 72 of file biasedcluster.cpp.
|
virtual |
Update the outputs of neurons.
Implements Updatable.
Definition at line 58 of file biasedcluster.cpp.
References OutputFunction::apply(), Cluster::inputs(), Cluster::outFunction(), Cluster::outputs(), and Cluster::setNeedReset().
The documentation for this class was generated from the following files:
- nnfw/include/biasedcluster.h
- nnfw/src/biasedcluster.cpp