The graphical representation of the MarXbot traction sensor. More...
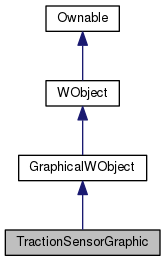
Public Member Functions | |
TractionSensorGraphic (WObject *object, const wVector &offset, real radius, real scale=1.0, real maxLength=1.0, real minLength=0.0, QString name="unamed") | |
Constructor. More... | |
~TractionSensorGraphic () | |
Destructor. More... | |
void | setVector (const wVector vector) |
Sets the vector to draw. The vector must be in the object frame of reference. More... | |
![]() | |
GraphicalWObject (World *world, QString name="unamed", const wMatrix &tm=wMatrix::identity()) | |
WObject * | attachedObject () const |
void | attachToObject (WObject *object, bool makeOwner=false, const wMatrix &displacement=wMatrix::identity()) |
const wMatrix & | getDisplacement () const |
void | setDisplacement (const wMatrix &displacement) |
void | updateAndCalculateAABB (wVector &minPoint, wVector &maxPoint, const wMatrix tm) |
void | updateAndCalculateOBB (wVector &dimension, wVector &minPoint, wVector &maxPoint) |
void | updateAndRender (RenderWObject *renderer, QGLContext *gw) |
void | updateAndRenderAABB (RenderWObject *renderer, RenderWorld *gw) |
![]() | |
WObject (World *world, QString name="unamed", const wMatrix &tm=wMatrix::identity(), bool addToWorld=true) | |
QColor | color () const |
void | drawLocalAxes (bool d) |
bool | isInvisible () const |
const QString & | label () const |
const QColor & | labelColor () const |
const wVector & | labelPosition () const |
bool | labelShown () const |
bool | localAxesDrawn () const |
const wMatrix & | matrix () const |
QString | name () const |
virtual void | postUpdate () |
virtual void | preUpdate () |
void | setAlpha (int alpha) |
void | setColor (QColor c) |
void | setInvisible (bool b) |
void | setLabel (QString label) |
void | setLabel (QString label, wVector pos, QColor color) |
void | setLabel (QString label, wVector pos) |
void | setLabelColor (const QColor &color) |
void | setLabelPosition (const wVector &pos) |
void | setMatrix (const wMatrix &newm) |
void | setPosition (real x, real y, real z) |
void | setPosition (const wVector &newpos) |
void | setTexture (QString textureName) |
void | setUseColorTextureOfOwner (bool b) |
void | showLabel (bool show) |
QString | texture () const |
bool | useColorTextureOfOwner () const |
const World * | world () const |
World * | world () |
![]() | |
const QList< Owned > & | owned () const |
Ownable * | owner () const |
void | setOwner (Ownable *owner, bool destroy=true) |
Protected Member Functions | |
void | drawArrow (wVector vector, real radius, real maxTipLength, QColor col, RenderWObject *renderer, QGLContext *gw) |
Draws an arrow. More... | |
real | limitComponent (real v, bool &limited) |
Limits the component to be between m_minLength and m_maxLength. More... | |
virtual void | render (RenderWObject *renderer, QGLContext *gw) |
Performs the actual drawing. More... | |
![]() | |
virtual void | calculateAABB (wVector &minPoint, wVector &maxPoint, const wMatrix tm) |
virtual void | calculateOBB (wVector &dimension, wVector &minPoint, wVector &maxPoint) |
virtual void | renderAABB (RenderWObject *renderer, RenderWorld *gw) |
void | updateMatrixFromAttachedObject () |
![]() | |
virtual void | changedMatrix () |
Protected Attributes | |
const real | m_maxLength |
The maximum length of the vector. More... | |
const real | m_maxTipLength |
The maximum length of the tip of the vector. More... | |
const real | m_minLength |
The minimum length of the vector. More... | |
WObject *const | m_object |
The object to which this representation is attached. More... | |
const real | m_radius |
The radius of the arrow. The length depends on the vector intensity. More... | |
const real | m_scale |
The scaling factor for the vector length. More... | |
wVector | m_vector |
The vector to represent. More... | |
QMutex | m_vectorMutex |
The mutex protecting the m_vector vector. More... | |
![]() | |
QColor | colorv |
bool | invisible |
QColor | labelcol |
bool | labeldrawn |
wVector | labelpos |
QString | labelv |
bool | localFrameOfReferenceDrawn |
QString | namev |
QString | texturev |
wMatrix | tm |
bool | usecolortextureofowner |
World * | worldv |
Additional Inherited Members | |
![]() | |
typedef QList< Owned > | OwnedList |
Detailed Description
The graphical representation of the MarXbot traction sensor.
The arrow is drawn as a cylinder with a cone on top
Definition at line 703 of file marxbotsensors.cpp.
Constructor & Destructor Documentation
|
inline |
Constructor.
This also sets a default color for the piece and sets handPiece to be our owner. This draws an arrow representing a vector
- Parameters
-
object the object to which this representation is attached. This must not be NULL offset the offset relative to object. The arrow starts from the offset in the frame of reference of the object radius the radius of the arrow. The length depends on the vector intensity scale the scaling factor for the vector length maxLength the maximum length of the vector in each axis. If the vector is longer than this on, the arrow is drawn this length with a different color. This is the length BEFORE scaling minLength the minimum length of the vector in each axis. If the vector is shorter than this, the arrow is not drawn. This is the length BEFORE scaling name the name of this object
Definition at line 730 of file marxbotsensors.cpp.
References GraphicalWObject::attachToObject(), wMatrix::identity(), TractionSensorGraphic::m_object, WObject::setTexture(), and WObject::setUseColorTextureOfOwner().
|
inline |
Destructor.
Definition at line 754 of file marxbotsensors.cpp.
Member Function Documentation
|
inlineprotected |
Draws an arrow.
This function can only be called by render(). This uses the current frame of reference
- Parameters
-
vector the vector to draw radius the radius of the arrow to draw maxTipLength the maximum length of the tip of the arrow col the color of the arrow renderer the RenderWObject object associated with this one. Use it e.g. to access the container gw the OpenGL context
Definition at line 823 of file marxbotsensors.cpp.
References WObject::color(), RenderWObject::container(), RenderWObjectContainer::drawCylinder(), wMatrix::grammSchmidt(), WObject::matrix(), farsa::min(), WObject::setColor(), and RenderWObjectContainer::setupColorTexture().
Referenced by TractionSensorGraphic::render().
Limits the component to be between m_minLength and m_maxLength.
This takes the sign of the component into account
- Parameters
-
v the value of the component to limit limited set to true if the value has been limited
- Returns
- the limited component
Definition at line 876 of file marxbotsensors.cpp.
References TractionSensorGraphic::m_maxLength, and TractionSensorGraphic::m_minLength.
Referenced by TractionSensorGraphic::render().
|
inlineprotectedvirtual |
Performs the actual drawing.
Draws the vector
- Parameters
-
renderer the RenderWObject object associated with this one. Use it e.g. to access the container gw the OpenGL context
Implements GraphicalWObject.
Definition at line 782 of file marxbotsensors.cpp.
References TractionSensorGraphic::drawArrow(), TractionSensorGraphic::limitComponent(), TractionSensorGraphic::m_maxTipLength, TractionSensorGraphic::m_radius, TractionSensorGraphic::m_scale, TractionSensorGraphic::m_vector, TractionSensorGraphic::m_vectorMutex, and WObject::tm.
|
inline |
Sets the vector to draw. The vector must be in the object frame of reference.
This function is thread-safe
- Parameters
-
vector the vector to draw. The vector must be in the object frame of reference
Definition at line 766 of file marxbotsensors.cpp.
References TractionSensorGraphic::m_vector, and TractionSensorGraphic::m_vectorMutex.
Referenced by MarXbotTractionSensor::update().
Member Data Documentation
|
protected |
The maximum length of the vector.
If the vector is longer than this, the arrow is drawn this length with a different color. This is the length BEFORE scaling
Definition at line 913 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::limitComponent().
|
protected |
The maximum length of the tip of the vector.
This is computed in the constructor
Definition at line 928 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::render().
|
protected |
The minimum length of the vector.
If the vector is shorter than this, the arrow is not drawn. This is the length BEFORE scaling
Definition at line 921 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::limitComponent().
|
protected |
The object to which this representation is attached.
Definition at line 893 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::TractionSensorGraphic().
|
protected |
The radius of the arrow. The length depends on the vector intensity.
Definition at line 899 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::render().
|
protected |
The scaling factor for the vector length.
Definition at line 904 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::render().
|
protected |
The vector to represent.
The vector is in the object frame of reference
Definition at line 935 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::render(), and TractionSensorGraphic::setVector().
|
protected |
The mutex protecting the m_vector vector.
The vector could be accessed by multiple threads concurrently, so we protect it with a mutex
Definition at line 943 of file marxbotsensors.cpp.
Referenced by TractionSensorGraphic::render(), and TractionSensorGraphic::setVector().
The documentation for this class was generated from the following file:
- experiments/src/marxbotsensors.cpp