The graphical representation of the linear camera. More...
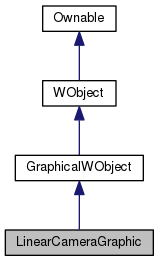
Public Member Functions | |
LinearCameraGraphic (WObject *object, const wMatrix &transformation, QVector< SimpleInterval > receptorsRanges, QString name="unamed") | |
Constructor. More... | |
~LinearCameraGraphic () | |
Destructor. More... | |
void | setPerceivedColors (const QVector< QColor > &receptors) |
Sets the colors perceived by the camera. More... | |
![]() | |
GraphicalWObject (World *world, QString name="unamed", const wMatrix &tm=wMatrix::identity()) | |
WObject * | attachedObject () const |
void | attachToObject (WObject *object, bool makeOwner=false, const wMatrix &displacement=wMatrix::identity()) |
const wMatrix & | getDisplacement () const |
void | setDisplacement (const wMatrix &displacement) |
void | updateAndCalculateAABB (wVector &minPoint, wVector &maxPoint, const wMatrix tm) |
void | updateAndCalculateOBB (wVector &dimension, wVector &minPoint, wVector &maxPoint) |
void | updateAndRender (RenderWObject *renderer, QGLContext *gw) |
void | updateAndRenderAABB (RenderWObject *renderer, RenderWorld *gw) |
![]() | |
WObject (World *world, QString name="unamed", const wMatrix &tm=wMatrix::identity(), bool addToWorld=true) | |
QColor | color () const |
void | drawLocalAxes (bool d) |
bool | isInvisible () const |
const QString & | label () const |
const QColor & | labelColor () const |
const wVector & | labelPosition () const |
bool | labelShown () const |
bool | localAxesDrawn () const |
const wMatrix & | matrix () const |
QString | name () const |
virtual void | postUpdate () |
virtual void | preUpdate () |
void | setAlpha (int alpha) |
void | setColor (QColor c) |
void | setInvisible (bool b) |
void | setLabel (QString label) |
void | setLabel (QString label, wVector pos, QColor color) |
void | setLabel (QString label, wVector pos) |
void | setLabelColor (const QColor &color) |
void | setLabelPosition (const wVector &pos) |
void | setMatrix (const wMatrix &newm) |
void | setPosition (real x, real y, real z) |
void | setPosition (const wVector &newpos) |
void | setTexture (QString textureName) |
void | setUseColorTextureOfOwner (bool b) |
void | showLabel (bool show) |
QString | texture () const |
bool | useColorTextureOfOwner () const |
const World * | world () const |
World * | world () |
![]() | |
const QList< Owned > & | owned () const |
Ownable * | owner () const |
void | setOwner (Ownable *owner, bool destroy=true) |
Protected Member Functions | |
virtual void | render (RenderWObject *renderer, QGLContext *gw) |
Performs the actual drawing. More... | |
![]() | |
virtual void | calculateAABB (wVector &minPoint, wVector &maxPoint, const wMatrix tm) |
virtual void | calculateOBB (wVector &dimension, wVector &minPoint, wVector &maxPoint) |
virtual void | renderAABB (RenderWObject *renderer, RenderWorld *gw) |
void | updateMatrixFromAttachedObject () |
![]() | |
virtual void | changedMatrix () |
Protected Attributes | |
WObject *const | m_object |
The object to which we are attached. More... | |
QVector< QColor > | m_receptors |
The vector with perceived colors. More... | |
QMutex | m_receptorsMutex |
The mutex protecting the m_receptors variable. More... | |
const QVector< SimpleInterval > | m_receptorsRanges |
The list of receptors. More... | |
![]() | |
QColor | colorv |
bool | invisible |
QColor | labelcol |
bool | labeldrawn |
wVector | labelpos |
QString | labelv |
bool | localFrameOfReferenceDrawn |
QString | namev |
QString | texturev |
wMatrix | tm |
bool | usecolortextureofowner |
World * | worldv |
Additional Inherited Members | |
![]() | |
typedef QList< Owned > | OwnedList |
Detailed Description
The graphical representation of the linear camera.
Definition at line 757 of file sensors.cpp.
Constructor & Destructor Documentation
|
inline |
Constructor.
This also sets the object to be our owner
- Parameters
-
object the object to which we are attached. transformation the transformation matrix relative the one of the object to which we are attached minAngle the minimum angle of the camera maxAngle the maximum angle of the camera numReceptors the number of receptors name the name of this object
Definition at line 773 of file sensors.cpp.
References GraphicalWObject::attachToObject(), LinearCameraGraphic::m_object, WObject::setColor(), WObject::setTexture(), and WObject::setUseColorTextureOfOwner().
|
inline |
Destructor.
Definition at line 792 of file sensors.cpp.
Member Function Documentation
|
inlineprotectedvirtual |
Performs the actual drawing.
- Parameters
-
renderer the RenderWObject object associated with this one. Use it e.g. to access the container gw the OpenGL context
Implements GraphicalWObject.
Definition at line 817 of file sensors.cpp.
References RenderWObject::container(), LinearCameraGraphic::m_receptors, LinearCameraGraphic::m_receptorsMutex, LinearCameraGraphic::m_receptorsRanges, RenderWObjectContainer::setupColorTexture(), and WObject::tm.
|
inline |
Sets the colors perceived by the camera.
This function is thread-safe
- Parameters
-
receptors the colors perceived by receptors
Definition at line 802 of file sensors.cpp.
References LinearCameraGraphic::m_receptors, and LinearCameraGraphic::m_receptorsMutex.
Referenced by LinearCamera::update().
Member Data Documentation
|
protected |
The object to which we are attached.
Definition at line 960 of file sensors.cpp.
Referenced by LinearCameraGraphic::LinearCameraGraphic().
|
protected |
The vector with perceived colors.
Definition at line 970 of file sensors.cpp.
Referenced by LinearCameraGraphic::render(), and LinearCameraGraphic::setPerceivedColors().
|
protected |
The mutex protecting the m_receptors variable.
The variable could be accessed by multiple threads concurrently, so we protect it with a mutex
Definition at line 978 of file sensors.cpp.
Referenced by LinearCameraGraphic::render(), and LinearCameraGraphic::setPerceivedColors().
|
protected |
The list of receptors.
Definition at line 965 of file sensors.cpp.
Referenced by LinearCameraGraphic::render().
The documentation for this class was generated from the following file:
- experiments/src/sensors.cpp