The class modelling the Khepera robot. More...
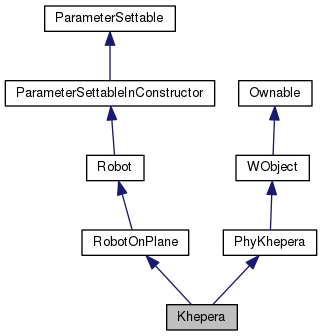
Public Member Functions | |
Khepera (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~Khepera () |
Destructor. More... | |
virtual bool | isKinematic () const |
Returns true if the robot is in kinematic mode. More... | |
virtual real | orientation (const Box2DWrapper *plane) const |
Returns the orientation of the robot. More... | |
virtual wVector | position () const |
Returns the position of the robot. More... | |
virtual QColor | robotColor () const |
Returns the color of the robot. More... | |
virtual real | robotHeight () const |
Returns the height of the robot. More... | |
virtual real | robotRadius () const |
Returns the radius of the robot. More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Saves the actual status of parameters into the ConfigurationParameters object passed. More... | |
virtual void | setOrientation (const Box2DWrapper *plane, real angle) |
Sets the orientation of the robot. More... | |
virtual void | setPosition (const Box2DWrapper *plane, real x, real y) |
Sets the position of the robot in the plane. More... | |
![]() | |
RobotOnPlane (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~RobotOnPlane () |
Destructor. More... | |
void | setPosition (const Box2DWrapper *plane, const wVector &pos) |
Sets the position of the robot in the plane. More... | |
![]() | |
Robot (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
virtual | ~Robot () |
Destructor. More... | |
![]() | |
ParameterSettableInConstructor (ConfigurationParameters &, QString) | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
virtual void | postConfigureInitialization () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
![]() | |
PhyKhepera (World *world, QString name, const wMatrix &transformation=wMatrix::identity()) | |
void | doKinematicSimulation (bool k) |
bool | getDrawFrontMarker () const |
bool | isKinematic () const |
virtual void | postUpdate () |
virtual void | preUpdate () |
SimulatedIRProximitySensorController * | proximityIRSensorController () |
void | setDrawFrontMarker (bool drawMarker) |
void | setProximityIRSensorsGraphicalProperties (bool drawSensor, bool drawRay=false, bool drawRealRay=false) |
WheelMotorController * | wheelsController () |
![]() | |
WObject (World *world, QString name="unamed", const wMatrix &tm=wMatrix::identity(), bool addToWorld=true) | |
QColor | color () const |
void | drawLocalAxes (bool d) |
bool | isInvisible () const |
const QString & | label () const |
const QColor & | labelColor () const |
const wVector & | labelPosition () const |
bool | labelShown () const |
bool | localAxesDrawn () const |
const wMatrix & | matrix () const |
QString | name () const |
void | setAlpha (int alpha) |
void | setColor (QColor c) |
void | setInvisible (bool b) |
void | setLabel (QString label) |
void | setLabel (QString label, wVector pos, QColor color) |
void | setLabel (QString label, wVector pos) |
void | setLabelColor (const QColor &color) |
void | setLabelPosition (const wVector &pos) |
void | setMatrix (const wMatrix &newm) |
void | setPosition (real x, real y, real z) |
void | setPosition (const wVector &newpos) |
void | setTexture (QString textureName) |
void | setUseColorTextureOfOwner (bool b) |
void | showLabel (bool show) |
QString | texture () const |
bool | useColorTextureOfOwner () const |
const World * | world () const |
World * | world () |
![]() | |
const QList< Owned > & | owned () const |
Ownable * | owner () const |
void | setOwner (Ownable *owner, bool destroy=true) |
Static Public Member Functions | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
typedef QList< Owned > | OwnedList |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
static const real | axletrack |
static const real | bodydistancefromground |
static const real | bodyh |
static const real | bodym |
static const real | bodyr |
static const real | passivewheelm |
static const real | passivewheelr |
static const real | wheelh |
static const real | wheelm |
static const real | wheelr |
![]() | |
void | setLeftWheelDesideredVelocity (real velocity) |
void | setRightWheelDesideredVelocity (real velocity) |
![]() | |
const QColor & | configuredRobotColor () const |
Returns the robot color specified by the configuration parameter. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
![]() | |
virtual void | changedMatrix () |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
![]() | |
QColor | colorv |
bool | invisible |
QColor | labelcol |
bool | labeldrawn |
wVector | labelpos |
QString | labelv |
bool | localFrameOfReferenceDrawn |
QString | namev |
QString | texturev |
wMatrix | tm |
bool | usecolortextureofowner |
World * | worldv |
Detailed Description
The class modelling the Khepera robot.
This inherits from PhyKhepera, so you can use all its methods
Constructor & Destructor Documentation
Khepera | ( | ConfigurationParameters & | params, |
QString | prefix | ||
) |
Constructor.
- Parameters
-
params the configuration parameters object with parameters to use prefix the prefix to use to access the object configuration parameters. This is guaranteed to end with the separator character when called by the factory, so you don't need to add one
Definition at line 368 of file robots.cpp.
References RobotOnPlane::configuredRobotColor(), PhyKhepera::doKinematicSimulation(), farsa::robotConfigurationUtilities::extractRobotName(), farsa::robotConfigurationUtilities::extractRobotTranformation(), farsa::robotConfigurationUtilities::extractWorld(), ConfigurationHelper::getBool(), PhyKhepera::proximityIRSensorController(), WObject::setColor(), PhyKhepera::setDrawFrontMarker(), SensorController::setEnabled(), MotorController::setEnabled(), PhyKhepera::setProximityIRSensorsGraphicalProperties(), and PhyKhepera::wheelsController().
|
virtual |
Destructor.
Definition at line 418 of file robots.cpp.
Member Function Documentation
|
static |
Add to Factory::typeDescriptions() the descriptions of all parameters and subgroups.
- Parameters
-
type is the name of the type regarding the description. The type is used when a subclass reuse the description of its parent calling the parent describe method passing the type of the subclass. In this way, the result of the method describe of the parent will be the addition of the description of the parameters of the parent class into the type of the subclass
Definition at line 395 of file robots.cpp.
References ParameterSettable::addTypeDescription(), and RobotOnPlane::describe().
|
virtual |
Returns true if the robot is in kinematic mode.
- Returns
- true if the robot is in kinematic mode
Implements RobotOnPlane.
Definition at line 459 of file robots.cpp.
References PhyKhepera::isKinematic().
|
virtual |
Returns the orientation of the robot.
This returns the angle around the z axis of the "plane". An angle of 0 means that the X axis of the plane and of the robot are coincident (positive angles follow the right-hand rule). This function returns the angle in radiants
- Parameters
-
plane the plane on which the robot is placed. You can use the plane returned by Arena::getPlane(), here.
- Warning
- the orientation returned by this function is not the same as the orientation set by setOrientation, there is a difference of (pi/2)
Implements RobotOnPlane.
Definition at line 443 of file robots.cpp.
References WObject::matrix(), and Box2DWrapper::phyObject().
|
virtual |
Returns the position of the robot.
- Returns
- the position of the robot
Implements RobotOnPlane.
Definition at line 428 of file robots.cpp.
References WObject::matrix().
Referenced by KheperaGroundSensor::update().
|
virtual |
Returns the color of the robot.
- Returns
- the color of the robot
Implements RobotOnPlane.
Definition at line 464 of file robots.cpp.
References WObject::color().
|
virtual |
Returns the height of the robot.
This is the height of the cylinder containing the robot. In some cases wheeled robots are modelled as cylinders to simplify calculations
- Returns
- the height of the robot
Implements RobotOnPlane.
Definition at line 449 of file robots.cpp.
References PhyKhepera::bodydistancefromground, and PhyKhepera::bodyh.
|
virtual |
Returns the radius of the robot.
This is the radius of the cylinder containing the robot. In some cases wheeled robots are modelled as cylinders to simplify calculations
- Returns
- the radius of the robot
Implements RobotOnPlane.
Definition at line 454 of file robots.cpp.
References PhyKhepera::bodyr.
|
virtual |
Saves the actual status of parameters into the ConfigurationParameters object passed.
This is not implemented, calling this causes an abort
- Parameters
-
params the configuration parameters object on which save actual parameters prefix the prefix to use to access the object configuration parameters.
Reimplemented from RobotOnPlane.
Definition at line 390 of file robots.cpp.
References Logger::error().
|
virtual |
Sets the orientation of the robot.
This modifies the angle around the z axis of the "plane". An angle of 0 means that the X axis of the plane and of the robot are coincident (positive angles follow the right-hand rule). This function wants the angle in radiants
- Parameters
-
plane the plane on which the robot is placed. You can use the plane returned by Arena::getPlane(), here. angle the new orientation in radiants
Implements RobotOnPlane.
Definition at line 433 of file robots.cpp.
References WObject::matrix(), and WObject::setMatrix().
|
virtual |
Sets the position of the robot in the plane.
The robot is placed on the face of the "plane" parallel to the local XY plane and with positive z coordinate.
- Parameters
-
plane the plane on which the robot should be placed. You can use the plane returned by Arena::getPlane(), here. x the new x coordinate y the new y coordinate
Implements RobotOnPlane.
Definition at line 422 of file robots.cpp.
References WObject::setPosition().
The documentation for this class was generated from the following files:
- experiments/include/robots.h
- experiments/src/robots.cpp