The base for classes that have a controllable flow of execution. More...
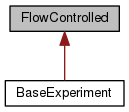
Public Member Functions | |
FlowControlled () | |
Constructor. More... | |
FlowControlled (FlowController *flowController) | |
Constructor. More... | |
void | setFlowController (FlowController *flowController) |
Sets the flow controller object to use. More... | |
Protected Member Functions | |
virtual void | flowControllerChanged (FlowController *flowController) |
The function called when the flow controller changes. More... | |
void | pauseFlow () |
Performs a pause in the if needed. More... | |
bool | stopFlow () |
Checks if execution should stop as soon as possible. More... | |
Detailed Description
The base for classes that have a controllable flow of execution.
This can be used as the base for classes that have a controllable flow of execution. Think, for example, of a robotic simulation: its execution can be implemented as a sequence of discrete steps. During each step the sensors are read, the motors are activated and the effects of the robot on the environment is computed. It is generally useful to provide who runs the simulation the possibility to stop it earlier and to control the delay between steps or to request the execution of one step externally. To implement these functionalities the simulation class can inherit from this class and use the stopFlow() and pauseFlow() functions at regular intervals. The former returns true when execution should be terminated, the latter pauses execution if needed, sleeps for a certain amount of time or returns immediately. Users of the simulation class, can then set the flow controller object (a subclass of FlowController) which is responsible of deciding when the simulation should stop or for how long it should sleep. Implementations of FlowController could, for example, check the status of a GUI which lets the user decide how to control the execution flow or can simply stop execution if it is taking too long. Using FlowControlled as a base for classes that implement long operations (possibly running in a separate thread) allows to only concentrate on when to check for stop requests and on how to separate the flow execution into steps (a step is what occurs between two subsequent calls to pauseFlow()), while leaving to other object the responsability to actually control the execution flow.
An example of an implementation of FlowControlled subclass can be as follows:
- Note
- When implementing a FlowController, make sure to also make the pause() function return when you want to stop execution. In fact, if the pause() function could wait for an external event (e.g. the user pressing a button), only having stop() return true could be not enough to actually stop execution (because the FlowControlled could be waiting on pauseFlow())
- This and FlowController are not thread-safe, you should make FlowController thread safe if you want to control execution of the FlowControlled subclass from a separate thread (e.g. when you have a simulation thread and a GUI thread)
Definition at line 170 of file flowcontrol.h.
Constructor & Destructor Documentation
|
inline |
Constructor.
This sets the flow controller to a DummyFlowController instance
Definition at line 178 of file flowcontrol.h.
|
inline |
Constructor.
This sets the flow controller to the given flow controller
- Parameters
-
flowController the flow controller object to use. If NULL, it sets the flow controller to an instance of DummyFlowController
Definition at line 192 of file flowcontrol.h.
Member Function Documentation
|
inlineprotectedvirtual |
The function called when the flow controller changes.
The default implementation does nothing. You can use this function to propagate the flow controller to subcomponents
- Parameters
-
flowController the new flow controller
Definition at line 248 of file flowcontrol.h.
|
inlineprotected |
Performs a pause in the if needed.
This function pauses, sleeps for a while or return immediately depending on the current flow controller
Definition at line 236 of file flowcontrol.h.
|
inline |
Sets the flow controller object to use.
The flow controller object is not deleted here,
- Parameters
-
flowController the flow controller object to use. If NULL, it sets the flow controller to an instance of DummyFlowController
Definition at line 206 of file flowcontrol.h.
Referenced by BaseExperiment::BaseExperiment().
|
inlineprotected |
Checks if execution should stop as soon as possible.
- Returns
- true if execution should stop as soon as possible
Definition at line 225 of file flowcontrol.h.
The documentation for this class was generated from the following file:
- experiments/include/flowcontrol.h