A class to add output neurons that can be used for custom operations. More...
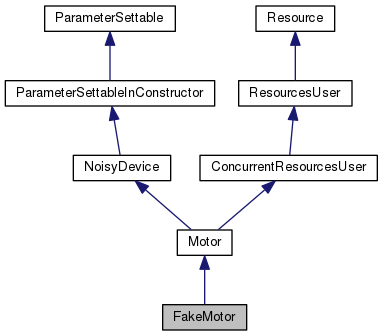
Public Member Functions | |
FakeMotor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor. More... | |
~FakeMotor () | |
Destructor. More... | |
virtual void | save (ConfigurationParameters ¶ms, QString prefix) |
Saves the parameters of the FakeMotor into the provided ConfigurationParameters object. More... | |
virtual void | shareResourcesWith (ResourcesUser *other) |
The function to share resources. More... | |
virtual int | size () |
Returns the number of outputs. More... | |
virtual void | update () |
Updates the state of the Motor every time step. More... | |
![]() | |
Motor (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~Motor () | |
Destructor. More... | |
QString | name () |
Return the name of the Sensor. More... | |
void | save (ConfigurationParameters ¶ms, QString prefix) |
Save the parameters into the ConfigurationParameters. More... | |
void | setName (QString name) |
Use this method for changing the name of the Sensor. More... | |
![]() | |
NoisyDevice (ConfigurationParameters ¶ms, QString prefix) | |
Constructor and Configure. More... | |
~NoisyDevice () | |
Destructor. More... | |
![]() | |
ParameterSettableInConstructor (ConfigurationParameters &, QString) | |
![]() | |
void | addObserver (RuntimeParameterObserver *obs) |
T | getRuntimeParameter (QString paramName) |
virtual ParameterSettableUI * | getUIManager () |
virtual void | postConfigureInitialization () |
void | removeObserver (RuntimeParameterObserver *obs) |
void | setRuntimeParameter (QString paramName, T newvalue) |
QString | typeName () const |
![]() | |
void | addUsableResource (QString resource) |
void | addUsableResources (QStringList resources) |
void | declareResource (QString name, T *resource, QString lockBuddy="") |
void | deleteResource (QString name) |
T * | getResource (QString name, bool *resourceExists=NULL) |
bool | hasResource (QString name) const |
void | removeAllUsableResources () |
void | removeUsableResource (QString resource) |
void | removeUsableResources (QStringList resources) |
void | usableResources (QStringList resources) |
bool | usedResourcesExist (QStringList *nonExistingResources=NULL) const |
Static Public Member Functions | |
static void | describe (QString type) |
Describes all the parameter needed to configure this class. More... | |
![]() | |
static void | describe (QString type) |
Describe all the parameter for configuring the Motor. More... | |
![]() | |
static void | describe (QString type) |
Describe all the parameter for configuring the Sensor. More... | |
![]() | |
static void | describe (QString type) |
static QString | fullParameterDescriptionPath (QString type, QString param) |
static QString | fullSubgroupDescriptionPath (QString type, QString sub) |
Protected Member Functions | |
void | resourceChanged (QString resourceName, ResourceChangeType changeType) |
The function called when a resource used here is changed. More... | |
![]() | |
QString | actualResourceNameForMultirobot (QString resourceName) const |
Returns the actual resource name to use. More... | |
void | checkAllNeededResourcesExist () |
Checks whether all resources we need are existing and throws an exception if they aren't. More... | |
void | resetNeededResourcesCheck () |
Resets the check on needed resources so that the next call to checkAllNeededResourcesExist() will perform the full check and not the quick one. More... | |
![]() | |
double | applyNoise (double v, double minValue, double maxValue) const |
Adds noise to the value. More... | |
![]() | |
void | notifyChangesToParam (QString paramName) |
![]() | |
T * | getResource () |
![]() | |
ResourcesUser (const ResourcesUser &other) | |
virtual void | notifyResourceChange (ResourceHandler *resource, ResourceChangeType changeType)=0 |
ResourcesUser & | operator= (const ResourcesUser &other) |
Protected Attributes | |
ResourceVector< real > | m_additionalOutputs |
The vector with additional outputs. More... | |
const QString | m_additionalOutputsResource |
The name of the resource associated with the vector of additional outputs. More... | |
NeuronsIterator * | m_neuronsIterator |
The object to iterate over neurons of the neural network. More... | |
const QString | m_neuronsIteratorResource |
The name of th resource associated with the neural network iterator. More... | |
![]() | |
ResourceCollectionHolder | m_resources |
Additional Inherited Members | |
![]() | |
enum | Property |
![]() | |
typedef Resource::ResourceChangeType | ResourceChangeType |
![]() | |
enum | ResourceChangeType |
![]() | |
AllowMultiple | |
Default | |
IsList | |
IsMandatory | |
![]() | |
static const double | Infinity |
static const int | MaxInteger |
static const int | MinInteger |
![]() | |
static Descriptor | addTypeDescription (QString type, QString shortHelp, QString longHelp=QString("")) |
static void | setGraphicalEditor (QString type) |
Detailed Description
A class to add output neurons that can be used for custom operations.
This class allows to add a given number of outputs to the controller and then provides a resource to access the value of the new neurons
In addition to all parameters defined by the parent class (Motor), this class also defines the following parameters:
- additionalOutputs: the number of outputs that will be added to the controller (default 1)
- neuronsIterator: the name of the resource associated with the neural network iterator (default is "neuronsIterator")
- additionalOutputsResource: the name of the resource that can be used to access the additional outputs (default is "additionalOutputs")
The resources required by this Motor are:
- name defined by the neuronsIterator parameter: the object to iterate over outputs of the controller
This motor also defines the following resources:
- name defined by the additionalOutputsResource parameter: the name of the resource that can be used to access the additional outputs. This can be accessed as a farsa::ResourceVector<real>
Constructor & Destructor Documentation
FakeMotor | ( | ConfigurationParameters & | params, |
QString | prefix | ||
) |
Constructor.
- Parameters
-
params the ConfigurationParameters containing the parameters prefix the path prefix to the paramters for this Motor
Definition at line 34 of file motors.cpp.
References FakeMotor::m_additionalOutputs, FakeMotor::m_additionalOutputsResource, FakeMotor::m_neuronsIteratorResource, ResourceVector< class >::size(), and ConcurrentResourcesUser::usableResources().
~FakeMotor | ( | ) |
Destructor.
Definition at line 48 of file motors.cpp.
References ConcurrentResourcesUser::deleteResource(), and FakeMotor::m_additionalOutputsResource.
Member Function Documentation
|
static |
Describes all the parameter needed to configure this class.
- Parameters
-
type a string representation for the name of this type
Definition at line 67 of file motors.cpp.
References ParameterSettable::addTypeDescription(), Motor::describe(), and ParameterSettable::IsMandatory.
|
protectedvirtual |
The function called when a resource used here is changed.
- Parameters
-
resourceName the name of the resource that has changed. chageType the type of change the resource has gone through (whether it was created, modified or deleted)
Reimplemented from ConcurrentResourcesUser.
Definition at line 105 of file motors.cpp.
References Logger::info(), FakeMotor::m_additionalOutputsResource, FakeMotor::m_neuronsIterator, FakeMotor::m_neuronsIteratorResource, Motor::name(), NeuronsIterator::nextNeuron(), Motor::resetNeededResourcesCheck(), NeuronsIterator::setCurrentBlock(), NeuronsIterator::setGraphicProperties(), and FakeMotor::size().
|
virtual |
Saves the parameters of the FakeMotor into the provided ConfigurationParameters object.
- Parameters
-
params the ConfigurationParameters where save the parameters prefix the path prefix for the parameters to save
Implements ParameterSettable.
Definition at line 58 of file motors.cpp.
References ConfigurationParameters::createParameter(), FakeMotor::m_additionalOutputs, FakeMotor::m_additionalOutputsResource, FakeMotor::m_neuronsIteratorResource, Motor::save(), ResourceVector< class >::size(), and ConfigurationParameters::startObjectParameters().
|
virtual |
The function to share resources.
The calling instance will lose the possibility to access the resources it had before this call
- Parameters
-
other the instance with which resources will be shared. If NULL we lose the association with other objects and start with an empty resource set
- Note
- This is NOT thread safe (both this and the other instance should not be being accessed by other threads)
Reimplemented from ConcurrentResourcesUser.
Definition at line 96 of file motors.cpp.
References ConcurrentResourcesUser::declareResource(), FakeMotor::m_additionalOutputs, FakeMotor::m_additionalOutputsResource, and ConcurrentResourcesUser::shareResourcesWith().
|
virtual |
Returns the number of outputs.
This returns the value set for the paramenter additionalOutputs
Implements Motor.
Definition at line 91 of file motors.cpp.
References FakeMotor::m_additionalOutputs, and ResourceVector< class >::size().
Referenced by FakeMotor::resourceChanged().
|
virtual |
Updates the state of the Motor every time step.
Implements Motor.
Definition at line 77 of file motors.cpp.
References Motor::checkAllNeededResourcesExist(), NeuronsIterator::getOutput(), FakeMotor::m_additionalOutputs, FakeMotor::m_neuronsIterator, Motor::name(), NeuronsIterator::nextNeuron(), NeuronsIterator::setCurrentBlock(), and ResourceVector< class >::size().
Member Data Documentation
|
protected |
The vector with additional outputs.
Definition at line 130 of file motors.h.
Referenced by FakeMotor::FakeMotor(), FakeMotor::save(), FakeMotor::shareResourcesWith(), FakeMotor::size(), and FakeMotor::update().
|
protected |
The name of the resource associated with the vector of additional outputs.
Definition at line 142 of file motors.h.
Referenced by FakeMotor::FakeMotor(), FakeMotor::resourceChanged(), FakeMotor::save(), FakeMotor::shareResourcesWith(), and FakeMotor::~FakeMotor().
|
protected |
The object to iterate over neurons of the neural network.
Definition at line 147 of file motors.h.
Referenced by FakeMotor::resourceChanged(), and FakeMotor::update().
|
protected |
The name of th resource associated with the neural network iterator.
Definition at line 136 of file motors.h.
Referenced by FakeMotor::FakeMotor(), FakeMotor::resourceChanged(), and FakeMotor::save().
The documentation for this class was generated from the following files:
- experiments/include/motors.h
- experiments/src/motors.cpp